JSON (JavaScript Object Notation) has become a player in the field of data exchange because of its straightforwardness and ease of understanding. Python, an used programming language offers strong capabilities for managing JSON data.
This piece explores the details of processing JSON data in Python with a focus on the json.load function and other related methods. We will discuss approaches, to converting JSON data into Python objects and back again aiming to provide a thorough grasp of these fundamental skills.
What Is JSON?
JSON, short for JavaScript Object Notation is a data format that is readable and writable, for humans and easy to process and create for machines. It relies on two structures:
- A set of name and value combinations, commonly known as an object, dictionary, hash table or associative array.
- A structured collection of values often known as an array, list or sequence.
Example of JSON:
Here’s an example of JSON data representing a simple object with name-value pairs and an array:
{
"name": "Alice",
"age": 30,
"is_student": false,
"courses": ["Math", "Science", "Art"]
}
In this example:
name
,age
, andis_student
are keys with corresponding values.courses
is a key with an array of values.
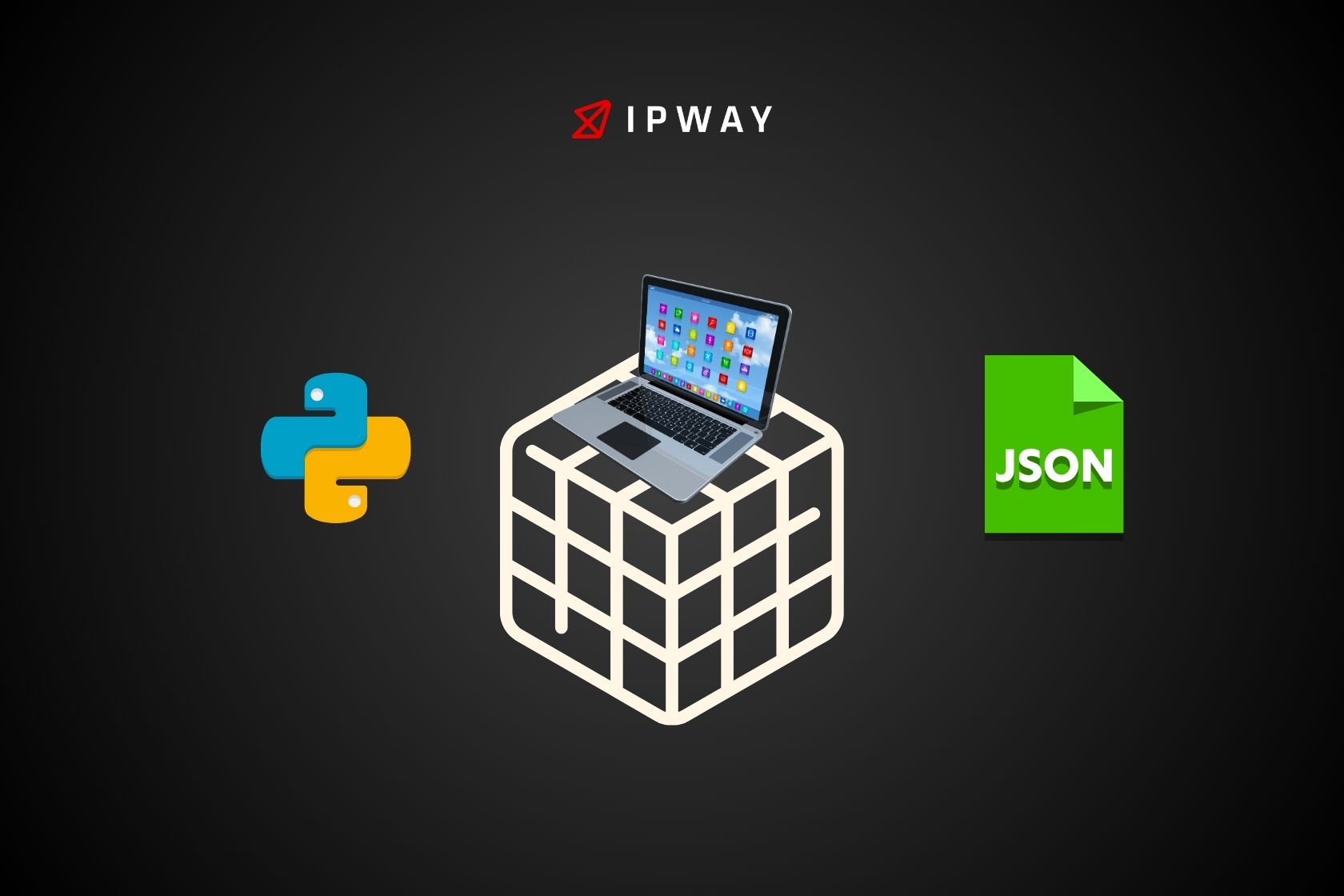
What is json.load?
The json.load function in Python is essential for handling JSON data saved in files. It helps convert a JSON file into a Python object, like a dictionary or list. Lets dive into how json.load functions and why it matters:
- Reading from a File: The primary function of json.load is to extract information directly from a file object. The file must be opened in read mode (‘r’). Must include correctly structured JSON data.
- Deserialization Process: Converting data into native Python data types is what deserialization entails. When json.load processes JSON data it links JSON objects to Python dictionaries, JSON arrays to Python lists JSON strings to Python strings and JSON numbers to Python integers or floats. This simplifies. Manipulating the data, in your Python code.
- Basic Usage:
- Open the file containing JSON data
- Use
json.load
to read the JSON data from the file and convert it into a Python object.
import json
# Sample JSON content in 'data.json':
# {
# "name": "Alice",
# "age": 30,
# "is_student": false,
# "courses": ["Math", "Science", "Art"]
# }
# Open the JSON file
with open('data.json', 'r') as file:
# Load JSON data into a Python object
data = json.load(file)
# Print the Python object
print(data) # Output: {'name': 'Alice', 'age': 30, 'is_student': False, 'courses': ['Math', 'Science', 'Art']}
4. Error Handling: When using json.load it’s important to address any errors, particularly the JSONDecodeError that may occur if the file contains invalid JSON. This helps your program effectively manage flawed or damaged JSON data.
import json
try:
with open('data.json', 'r') as file:
data = json.load(file)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
5. Optional Parameters:
- cls: Describes a JSON decoding class. By default JSONDecoder is employed.
- object_hook: A feature that can convert the JSON data into a Python object. This function is invoked for each decoded JSON object.
- parse_float: When required it will be referred to with the string of each decimal number, for decoding.
- parse_int: If needed it will be referred to as the string containing each JSON integer, for decoding.
- parse_constant: If required it will be referred to by one of the following terms; ‘NaN’ ‘Infinity’ or’ Infinity’.
import json
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def decode_person(dct):
if "name" in dct and "age" in dct:
return Person(dct["name"], dct["age"])
return dct
with open('data.json', 'r') as file:
person = json.load(file, object_hook=decode_person)
print(person.__dict__)
By grasping the concept and using the json.load method you can effectively. Interpret JSON data from files making it easier to exchange and work with data in your Python programs. This method comes in handy when dealing with configuration files data formats, for sharing information or JSON formatted API responses.
Converting JSON string to Python object
When dealing with data exchange formats it’s quite common to convert a JSON string into a Python object. In Python you can accomplish this by using the json.loads() function found in the json module. Let me walk you through how this procedure unfolds step, by step:
Import the JSON Module: Before you can use json.loads()
, you need to import the json
module.
import json
JSON String: Make sure you have your JSON string prepared. The string should follow the JSON format and be enclosed within double quotation marks.
json_string = '{"name": "Alice", "age": 30, "is_student": false, "courses": ["Math", "Science", "Art"]}'
Use json.loads()
: This feature reads through the JSON string. Transforms it into a Python dictionary or other suitable data structures, in Python.
Output: The Python object that is generated can be utilized similarly to a dictionary or list, in Python.
print(python_obj)
# Output: {'name': 'Alice', 'age': 30, 'is_student': False, 'courses': ['Math', 'Science', 'Art']}
Example in Detail
Think about a JSON string that shows a users profile. This string contains types of data, like text, numbers, true/false values and lists.
Step-by-Step Example:
json_string = '''
{
"username": "john_doe",
"active": true,
"email": "[email protected]",
"roles": ["admin", "user"],
"age": 28
}
'''
Convert to Python Object
import json
# Parsing JSON string
user_profile = json.loads(json_string)
Accessing Data
print(user_profile["username"]) # Output: john_doe
print(user_profile["active"]) # Output: True
print(user_profile["email"]) # Output: [email protected]
print(user_profile["roles"]) # Output: ['admin', 'user']
print(user_profile["age"]) # Output: 28
Handling Errors
Dealing with errors that may arise during the conversion process is crucial. One typical issue is encountering a json.JSONDecodeError signaling that the JSON string lacks formatting.
try:
python_obj = json.loads(json_string)
except json.JSONDecodeError as e:
print(f"Error decoding JSON: {e}")
Converting JSON file to Python object
Dealing with errors that may arise during the conversion process is crucial. One typical issue is encountering a json.JSONDecodeError signaling that the JSON string lacks formatting.
Converting a Python object to a JSON string is a process known as serialization or JSON encoding. This is typically done using the json.dumps()
function from the json
module. Here’s a detailed breakdown of how this conversion works:
Import the JSON Module: Before using json.dumps()
, import the json
module.
import json
Python Object: Prepare your Python object, which could be a dictionary, list, tuple, string, etc.
python_obj = {
"name": "Alice",
"age": 30,
"is_student": False,
"courses": ["Math", "Science", "Art"]
}
Use json.dumps()
: This function serializes the Python object into a JSON-formatted string.
json_string = json.dumps(python_obj)
Output: The resulting JSON string can be printed or used for data exchange.
print(json_string)
# Output: '{"name": "Alice", "age": 30, "is_student": false, "courses": ["Math", "Science", "Art"]}'
Example in Detail
Python Dictionary:
book = {
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"published_year": 1960,
"genres": ["Fiction", "Drama"]
}
Convert to JSON String:
import json
# Serializing Python object to JSON string
json_string = json.dumps(book)
Formatted Output: To make the JSON string more readable, you can use the indent
parameter to add indentation.
json_string = json.dumps(book, indent=4)
print(json_string)
Handling Complex Objects
For custom Python objects, you need to define a custom encoder by subclassing json.JSONEncoder
:
class Book:
def __init__(self, title, author, year, genres):
self.title = title
self.author = author
self.year = year
self.genres = genres
class BookEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Book):
return obj.__dict__
return super().default(obj)
book = Book("To Kill a Mockingbird", "Harper Lee", 1960, ["Fiction", "Drama"])
json_string = json.dumps(book, cls=BookEncoder)
print(json_string)
When you change Python elements into JSON text you enable the transfer of information between systems or parts simplifying the storage, transmission and handling of data in a standardized way. This step is crucial, for programs that engage with services, APIs or setup files.
Writing Python object to a JSON file
Converting a Python object into a JSON file is referred to as serialization or encoding. To achieve this you can utilize the json.dump() function found in the json module. Let me walk you through the steps involved in completing this process:
Import the JSON Module: Start by importing the json
module.
import json
Prepare the Python Object: This object can be a dictionary, list, or any serializable Python data structure.
python_obj = {
"name": "Alice",
"age": 30,
"is_student": False,
"courses": ["Math", "Science", "Art"]
}
Use json.dump()
: This function serializes the Python object and writes it to a file.
with open('data.json', 'w') as file:
json.dump(python_obj, file)
Adding Indentation: To enhance the readability of the JSON file you can improve its format by incorporating indentation through the utilization of the parameter.
with open('data.json', 'w') as file:
json.dump(python_obj, file, indent=4)
Example in Detail
Step-by-Step Example:
Python Dictionary:
book = {
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"published_year": 1960,
"genres": ["Fiction", "Drama"]
}
Write to JSON File:
import json
# Open the file in write mode
with open('book.json', 'w') as file:
# Serialize the Python object to JSON and write to the file
json.dump(book, file)
Formatted Output:
with open('book.json', 'w') as file:
json.dump(book, file, indent=4)
Handling Custom Objects
When creating Python objects you must craft a personalized encoder by extending json.JSONEncoder.
class Book:
def __init__(self, title, author, year, genres):
self.title = title
self.author = author
self.year = year
self.genres = genres
class BookEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Book):
return obj.__dict__
return super().default(obj)
book = Book("To Kill a Mockingbird", "Harper Lee", 1960, ["Fiction", "Drama"])
with open('book.json', 'w') as file:
json.dump(book, file, cls=BookEncoder, indent=4)
Storing and sharing data in a format that is readable by both humans and machines can be done effortlessly by saving Python objects to a JSON file. This method comes in handy for tasks like preserving configurations ensuring data persistence and facilitating data transfer, among systems or components.
Converting Custom Python Objects to JSON Objects
When you want to convert your Python objects into JSON format you’ll need to follow a special serialization method because the standard JSON encoder doesn’t support custom classes. Below is an, in depth explanation of how you can make this conversion:
Define a Custom Class: Create a Python class for your custom objects.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Create a Custom JSON Encoder: Extend the json.JSONEncoder. Customize the default method to define the conversion of the custom object into a format compatible, with JSON.
import json
class PersonEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Person):
return obj.__dict__
return super().default(obj)
Serialize Custom Object to JSON: You can employ json.dumps() alongside an encoder to transform the customized object into a JSON string.
person = Person("Alice", 30)
json_string = json.dumps(person, cls=PersonEncoder)
print(json_string) # Output: {"name": "Alice", "age": 30}
If you have a Book class and need to change the instances of this class into JSON objects.
Define the Custom Class:
class Book:
def __init__(self, title, author, published_year, genres):
self.title = title
self.author = author
self.published_year = published_year
self.genres = genres
Create the Custom JSON Encoder:
class BookEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Book):
return {
"title": obj.title,
"author": obj.author,
"published_year": obj.published_year,
"genres": obj.genres
}
return super().default(obj)
Serialize the Custom Object:
book = Book("To Kill a Mockingbird", "Harper Lee", 1960, ["Fiction", "Drama"])
json_string = json.dumps(book, cls=BookEncoder, indent=4)
print(json_string)
Handling Nested Custom Objects
If your personalized items include personalized items it’s important to make sure that the encoder can manage these nested objects too.
class Publisher:
def __init__(self, name, founded):
self.name = name
self.founded = founded
class Book:
def __init__(self, title, author, published_year, genres, publisher):
self.title = title
self.author = author
self.published_year = published_year
self.genres = genres
self.publisher = publisher
class ComplexEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, Book):
return {
"title": obj.title,
"author": obj.author,
"published_year": obj.published_year,
"genres": obj.genres,
"publisher": obj.publisher.__dict__
}
if isinstance(obj, Publisher):
return obj.__dict__
return super().default(obj)
publisher = Publisher("J.B. Lippincott & Co.", 1836)
book = Book("To Kill a Mockingbird", "Harper Lee", 1960, ["Fiction", "Drama"], publisher)
json_string = json.dumps(book, cls=ComplexEncoder, indent=4)
print(json_string)
Creating Python class objects from JSON objects
Converting JSON data back into Python objects also known as deserialization involves the process of creating Python class objects from objects. This can be done by utilizing the json.loads() function along, with the parameter, which enables customized handling of JSON objects.
Define the Custom Class: Create a Python class to represent your custom objects
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Define a Function for Object Hook: This function will convert dictionaries to instances of the custom class.
def decode_person(dct):
if "name" in dct and "age" in dct:
return Person(dct["name"], dct["age"])
return dct
Use json.loads()
with object_hook
: Deserialize the JSON string into a custom Python object.
import json
json_string = '{"name": "Alice", "age": 30}'
person = json.loads(json_string, object_hook=decode_person)
print(person.name) # Output: Alice
print(person.age) # Output: 30
Imagine a situation where you come across a JSON string that describes a book and you wish to transform this information into an object of a Book class.
Define the Custom Class:
class Book:
def __init__(self, title, author, published_year, genres):
self.title = title
self.author = author
self.published_year = published_year
self.genres = genres
Define the Object Hook Function:
def decode_book(dct):
if "title" in dct and "author" in dct and "published_year" in dct and "genres" in dct:
return Book(dct["title"], dct["author"], dct["published_year"], dct["genres"])
return dct
Deserialize the JSON String:
json_string = '''
{
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"published_year": 1960,
"genres": ["Fiction", "Drama"]
}
'''
book = json.loads(json_string, object_hook=decode_book)
print(book.title) # Output: To Kill a Mockingbird
print(book.author) # Output: Harper Lee
print(book.published_year) # Output: 1960
print(book.genres) # Output: ['Fiction', 'Drama']
Handling Nested Custom Objects
When dealing with JSON data containing custom objects it is crucial to make sure that the object hook function is capable of managing the nested structures effectively.
class Publisher:
def __init__(self, name, founded):
self.name = name
self.founded = founded
class Book:
def __init__(self, title, author, published_year, genres, publisher):
self.title = title
self.author = author
self.published_year = published_year
self.genres = genres
self.publisher = publisher
def decode_complex(dct):
if "name" in dct and "founded" in dct:
return Publisher(dct["name"], dct["founded"])
if "title" in dct and "author" in dct and "published_year" in dct and "genres" in dct and "publisher" in dct:
publisher = decode_complex(dct["publisher"])
return Book(dct["title"], dct["author"], dct["published_year"], dct["genres"], publisher)
return dct
json_string = '''
{
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"published_year": 1960,
"genres": ["Fiction", "Drama"],
"publisher": {
"name": "J.B. Lippincott & Co.",
"founded": 1836
}
}
'''
book = json.loads(json_string, object_hook=decode_complex)
print(book.title) # Output: To Kill a Mockingbird
print(book.publisher.name) # Output: J.B. Lippincott & Co.
Loading vs dumping
The Python json module includes four functions: dump(), dumps() load() and loads(). It can get tricky to distinguish between them. One helpful trick is to associate the letter ‘S’ with “String.” Hence you can link loads() to “load-s” (loading from a string).
Dumps() to “dump-s” (dumping into a string). This clarification makes it easier to understand that load() and dump() work with file objects whereas loads() and dumps() deal with string operations.
Conclusion
Working with JSON data in Python is crucial for a range of tasks particularly when interacting with APIs and web services. Understanding how to utilize functions like json.load, json.loads, json.dump and json.dumps well as creating custom encoders and decoders allows you to effectively manage and modify JSON data.
This guide serves as a starting point, for navigating JSON in Python enabling you to confidently tackle tasks related to data serialization and deserialization.
Discover how IPWAY’s innovative solutions can revolutionize your parsing experience for a better and more efficient approach.