When it comes to moving data and fetching information from the web, cURL (Client URL) is a crucial tool. It’s a command line tool that helps in exchanging data with servers through different protocols, like HTTP, HTTPS, FTP and more.
One of its functions is downloading files. In this guide we’ll delve into the effective ways of how to download file with cURL discussing different methods and recommended approaches.
Managing file names
When you use cURL to download a file it’s important to handle file names for organization and automation. By default cURL shows the output directly in the terminal. To save the output to a file you have to indicate the o or output option along, with the name of the file you want.
curl -o filename.ext http://example.com/file.ext
To download a file from an URL and save it specify the filename.ext. If you prefer the saved file to have the name as the original file on the server opt, for the O or remote name choice:
curl -O http://example.com/file.ext
This will save the file as file.ext
, matching the name from the server.
Handling redirects
Web servers commonly redirect requests to alternative URLs. To make sure you’re correctly navigating these redirects and accessing the desired file utilize the L or location option.
curl -L -o filename.ext http://example.com/redirect
When you use this command with cURL it instructs the program to track and go through any redirects until it lands on the destination URL and then proceed to download the file.
Download file with cURL and multiple files
cURL is quite handy when it comes to downloading files all at once with just one command, which makes it really efficient, for batch downloads. Simply list the URLs you want to download making sure to separate them with spaces.
curl -O http://example.com/file1.ext -O http://example.com/file2.ext
Alternatively, you can use a file containing a list of URLs and the -K
or --config
option:
curl -K urls.txt
Where urls.txt
contains:
http://example.com/file1.ext
http://example.com/file2.ext
Rate limiting
When you’re grabbing files, from online servers it’s crucial to think about rate limiting to prevent the server from getting swamped and to follow usage guidelines. CURL offers the limit rate feature, for managing the download speed.
curl --limit-rate 100K -O http://example.com/file.ext
This command limits the download speed to 100 KB/s.
Silent Downloads
By default when using cURL progress updates are displayed in the terminal.. There could be times when you’d prefer to hide this information especially in automated scripts. You can achieve this by utilizing the s or silent flag, for downloads.
curl -s -O http://example.com/file.ext
For a completely silent mode that also suppresses error messages, use -sS
:
curl -sS -O http://example.com/file.ext
Authentication
Certain servers need authentication for file access. CURL offers authentication methods like Basic, Digest and NTLM. To implement authentication utilize the u or user option, with the username and password specified afterwards.
curl -u username:password -O http://example.com/file.ext
For security, in authentication check out the cURL documentation.
Download Through Proxy
To download files through a proxy server, use the -x
or --proxy
option:
curl -x proxyserver:port -O http://example.com/file.ext
If the proxy requires authentication, use the --proxy-user
option:
curl -x proxyserver:port --proxy-user username:password -O http://example.com/file.ext
Download Progress
Keeping track of the download status is crucial when dealing with files. The cURL tool provides a feature, the # option to show a progress bar:
curl -# -O http://example.com/largefile.ext
For more detailed information, use the -w
or --write-out
option to format output:
curl -w "Downloaded %{size_download} bytes in %{time_total} seconds" -O http://example.com/largefile.ext
Request/Response Information
To debug or get more details about the request and response, use the -v
or --verbose
option:
curl -v -O http://example.com/file.ext
For a more concise output, use the -I
or --head
option to fetch only the HTTP headers:
curl -I http://example.com/file.ext
cURL vs. Wget
Downloading files from the internet involves using two used command line tools; cURL and Wget. While both are efficient and popular they excel in areas and are tailored for specific purposes. In this segment we’ll delve into a comparison of cURL and Wget exploring their characteristics, applications and benefits to assist you in determining the most suitable tool, for your requirements.
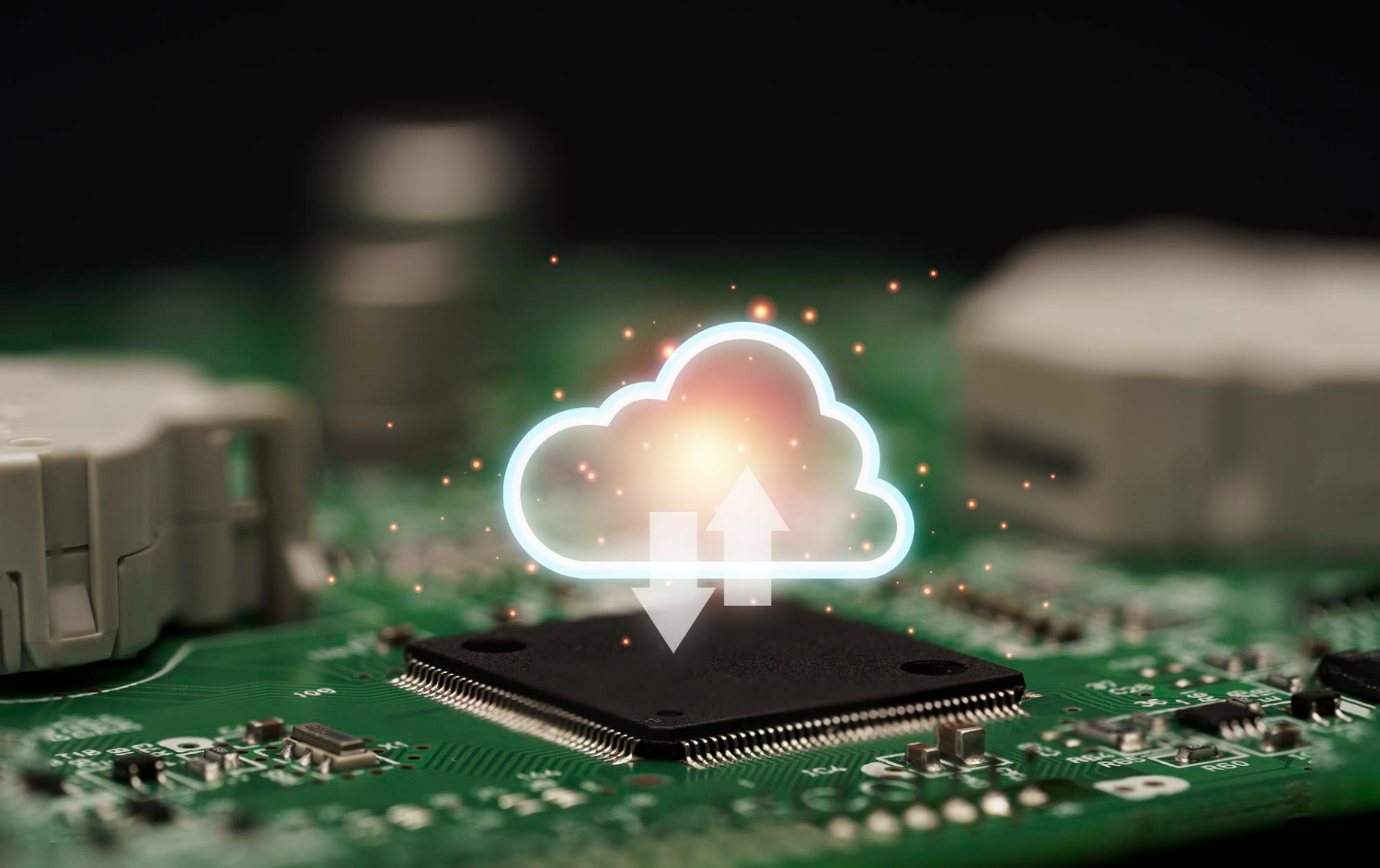
Overview of cURL
cURL, short for “Client URL ” is a command line tool created to move data to or from a server through different protocols like HTTP, HTTPS, FTP and others. Its adaptability and precise management of network requests have made it popular, among developers and system administrators.
Key Features of cURL
Protocol Support: cURL, short for “Client URL ” is a command line tool created to move data to or from a server through different protocols like HTTP, HTTPS, FTP and others. Its adaptability and precise management of network requests have made it popular, among developers and system administrators.
Granular Control: Users have the ability to manage aspects of a network request using cURL, such, as customizing headers selecting request methods and handling cookies.
Authentication: cURL offers a range of authentication options such as Basic, Digest, NTLM, Negotiate and OAuth to ensure access, to protected resources.
Data Manipulation: cURL is great for managing data operations like encoding URLs compressing data and submitting forms. It’s perfect, for engaging with APIs and online services.
Scripting and Automation: cURL is very adaptable. Can be included in shell scripts and automation processes which makes it a valuable tool, for automating data transfers.
Overview of Wget
Wget, which stands for “World Web get ” is a tool used through the command line that is mainly meant for fetching files from the internet. It is recognized for its straightforwardness, reliability and capability to conduct downloads making it a great option, for duplicating websites and downloading extensive collections of files.
Key Features of Wget
Recursive Downloads: Wget is great at fetching websites or directories by navigating links in a recursive manner. This functionality proves handy for tasks, like web scraping and duplicating websites.
Retry Mechanism: Wget comes with a feature that allows it to retry failed downloads automatically making sure that files are successfully downloaded when there are network problems.
Resume Downloads: When using Wget you can resume downloads if they get interrupted, especially useful for getting files, on shaky connections.
Mirroring and Timestamping: You can use Wget to replicate websites while maintaining the folder arrangement and file timestamps simplifying the process of keeping website copies current.
User-Friendly: Wget is made to be user friendly and easy to use, with commands and options that help with basic downloading tasks.
Common Mistakes and Best Practices
When you use cURL to download files it’s important to be cautious to prevent errors, downloads or unexpected outcomes. Knowing the mistakes and following recommended methods will help you use cURL properly and safely. In this guide we’ll explore the errors users face and provide tips, on how to steer clear of them.
Common Mistakes
Not Following Redirects
- Mistake: Usually when you’re using cURL to download a file from a URL that redirects to another location cURL won’t automatically follow those redirects. This means that if you attempt to download a file from a URL that redirects elsewhere cURL may not successfully grab the file you intended to download.
- Solution: Make sure to include the location flag when using cURL so that it can correctly navigate through redirects until it reaches the final destination.
curl -L -O http://example.com/redirected-file
Incorrect File Naming
- Mistake: Users frequently forget to mention the name of the output file, which leads to the data showing up in the terminal of being stored in a file.
- Solution: Use the
-o
or--output
option to specify the desired file name.
curl -o outputfile.ext http://example.com/file.ext
- If you want to save the file with the same name as on the server, use
-O
or--remote-name
.
curl -O http://example.com/file.ext
Ignoring Rate Limits
- Mistake: Downloading files at a pace may cause strain, on the server potentially resulting in throttling or IP bans.
- Solution: Use the
--limit-rate
option to control the download speed and avoid overloading the server.
curl --limit-rate 100K -O http://example.com/file.ext
Silent Failures
- Mistake: When using cURL in mode ( s or silent) it’s important to include proper error handling to avoid overlooking any potential failures.
- Solution: Use
-sS
to run cURL silently but still show error messages.
curl -sS -O http://example.com/file.ext
Ignoring SSL/TLS Verification
- Mistake: Using SSL/TLS verification ( k or insecure) without knowing the security risks involved could make you vulnerable to Man, in the Middle attacks.
- Solution: Make sure to verify SSL/TLS correctly unless its absolutely required and even then be aware of the potential risks.
Best Practices
Use Verbose Mode for Debugging
- When you’re trying to troubleshoot make sure to utilize the v or verbose option for an in depth look, at the request and response details.
curl -v -O http://example.com/file.ext
Handle Redirects Properly
- Always include the
-L
option to ensure all redirects are followed until the final URL is reached.
curl -L -O http://example.com/redirected-file.ext
Manage Output Files Carefully
- Use the
-o
option to specify output file names explicitly and-O
to use the remote file name.
curl -o myfile.ext http://example.com/file.ext
curl -O http://example.com/file.ext
Implement Rate Limiting
- Use the
--limit-rate
option to prevent server overload and adhere to server policies.
curl --limit-rate 200K -O http://example.com/largefile.ext
Authenticate Securely
- Use the appropriate authentication method and keep credentials secure. For basic authentication, use:
curl -u username:password -O http://example.com/securefile.ext
Check SSL/TLS Certificates
- Ensure SSL/TLS certificates are verified to maintain secure connections.
curl --cert /path/to/cert.pem --key /path/to/key.pem -O https://secure.example.com/file.ext
Use Configuration Files for Complex Requests
- For tasks that’re intricate or need to be done repeatedly you can utilize a configuration file and include the K option to save your cURL commands.
curl -K config.txt
- Example
config.txt
content:
url = "http://example.com/file.ext"
output = "outputfile.ext"
limit-rate = "100K"
Monitor and Log Downloads
- Remember to keep a record of your downloads for problem solving purposes and to confirm that transfers have been successful. Utilize the w feature, to structure output and store it in a log file.
Conclusion
Downloading file with cURL is a robust method for handling file transfers. This guide covers options and strategies to help you enhance your download procedures adhere to server regulations and manage files effectively. Whether you’re fetching a file or overseeing extensive data transfers cURL offers the necessary resources, for accomplishing tasks efficiently.
Discover how IPWAY’s innovative solutions can revolutionize your web scraping experience for a better and more efficient approach.