In todays era data holds great importance. Whether you’re a programmer, scholar or cinema lover having access to amounts of data can offer valuable perspectives. One of the comprehensive sources of movie related details is IMDb. In this 2024 manual we will guide you through the steps of gathering IMDb data starting from configuring your setup to retrieving movie details and reviews. We will also delve into how to export the collected data into JSON and CSV formats for analysis and utilization.
IMDb, known as the Internet Movie Database stands as a crucial reference for individuals interested in movies, television programs and the entertainment sector. It harbors information about movies, actors, production teams and more. By collecting IMDb data through scraping techniques you can unveil a wealth of insights, for academic studies, personal endeavors or enhancing your business.
Why Scrape IMDb Data?
Mining information from IMDb can be beneficial, for a range of reasons. Lets explore some motivations behind this practice:
Gain insights into movie trends and popularity: By examining information, from IMDb one can spot patterns in the types of movies the popularity of actors and how well films do at the box office.
Analyze movie reviews and ratings: Analyzing the feedback and ratings from viewers can provide insights, for studying films developing marketing plans and enhancing audience involvement.
Build movie recommendation systems: Utilize information from IMDb data to suggest movies tailored to tastes and viewing habits.
Conduct academic research on film studies: Scholars have the opportunity to delve into film history track the evolution of genres and explore the influences by utilizing data, from IMDb.
Enhance your personal movie database: For those who love movies collecting information, from IMDb can be a way to keep your personal movie collection current and detailed.
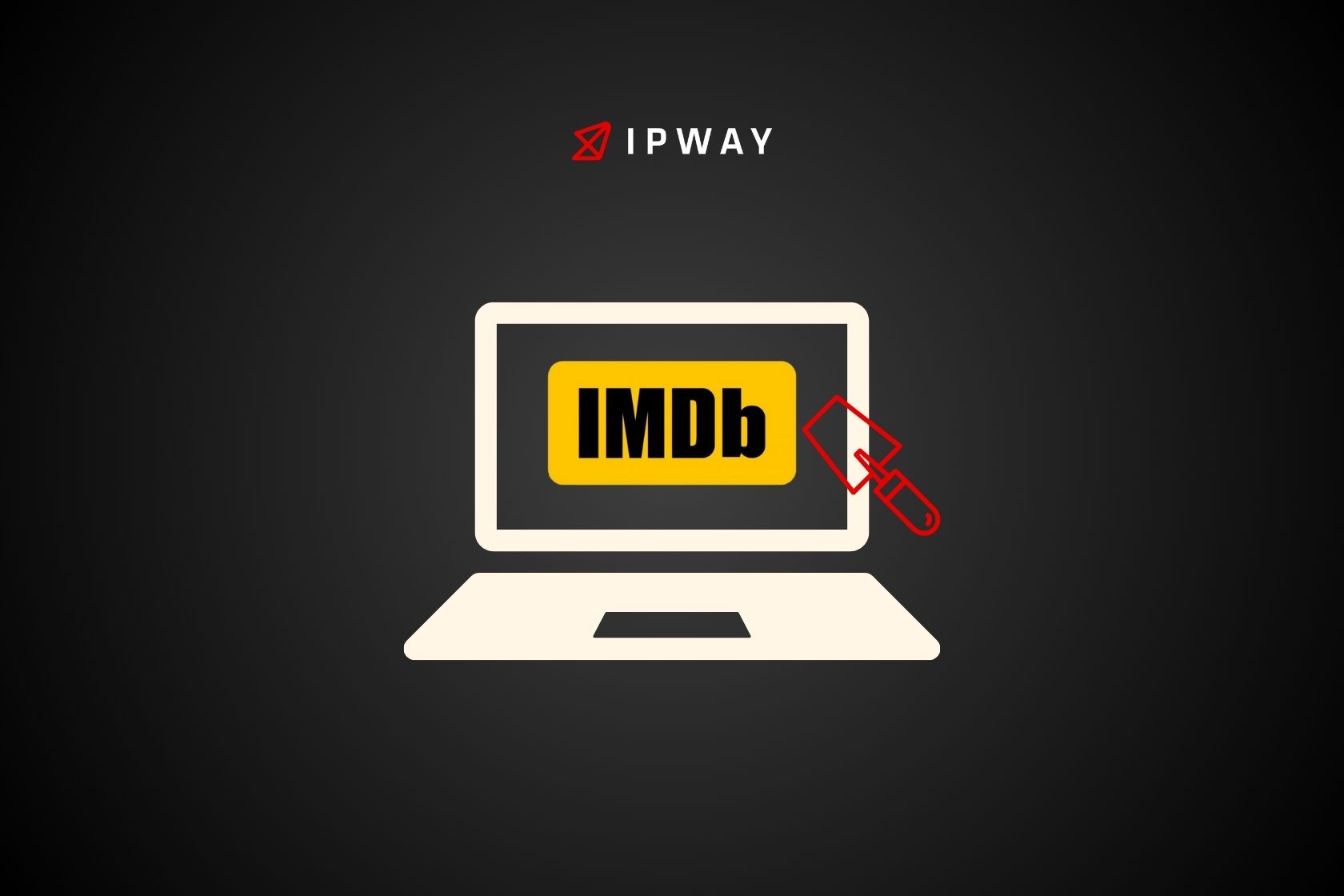
1. Setting Up for Scraping IMDb
Before starting to extract IMDb data, make sure to prepare your workspace and verify that you have all the tools. Here’s a guide to kick off the process:
Choose Your Programming Language and Tools:
- Python: Python stands out as an option for web scraping because of its ease of use and the availability of robust libraries such, as BeautifulSoup, Scrapy and Selenium.
- JavaScript: You can also utilize Node.js along with tools such as Puppeteer, for web scraping particularly when handling websites that heavily rely on JavaScript.
Install Necessary Libraries: Make sure you’ve got all the necessary libraries installed. Use pip to install them in Python.
pip install requests
pip install beautifulsoup4
pip install scrapy
pip install selenium
Set Up a Virtual Environment: Setting up a space can assist in handling dependencies and preventing clashes.
python -m venv scraping_env
source scraping_env/bin/activate
Understand IMDb’s Structure: Get to know the layout of IMDbs website so you can find the HTML elements that hold the information you’re looking for. Utilize your browsers Developer Tools (right click on the page and choose “Inspect”) to check out the HTML and CSS.
Respect IMDb’s Terms of Service: Make sure to check IMDbs terms of service before scraping data. It’s important to follow their guidelines to stay in compliance and avoid any complications.
2. Overview of Web Scraper API
Utilizing a Web Scraper API can make the extraction of IMDb information. These APIs manage challenges associated with web scraping like rotating IP addresses and dealing with CAPTCHAs.
What is a Web Scraper API?
A tool that offers a way to pull information from websites without dealing with the technical details of web scraping. It simplifies the process. Provides a reliable solution.
Popular Web Scraper APIs
ScraperAPI: A tool that handles proxies and resolves CAPTCHAs on your behalf enabling you to concentrate on gathering information.
Octoparse: A tool for extracting data without the need for coding, designed for individuals who are not proficient, in programming.
Scrapy Cloud: A service, for launching and operating Scrapy spiders providing scalability and simple administration.
Benefits of Using a Web Scraper API
Improved Efficiency: Using APIs can help with backend functions like handling IPs and solving CAPTCHAs, which ultimately speeds up and streamlines your web scraping activities.
Reduced Risk of IP Blocking: APIs commonly incorporate IP rotation and proxy management to lower the risk of your IP address getting blocked.
Easier Data Extraction: APIs offer organized and tidy data, available in formats such, as JSON or CSV all set for analysis.
3. Scraping movie info from a list
Now that you’ve arranged your workspace and selected your tools lets explore extracting movie details from IMDb. We’ll concentrate on gathering information from the 250 movies list, on IMDb as an illustration.
Identify the Target URL: For this example, we’ll scrape data from IMDb’s top 250 movies list: https://www.imdb.com/chart/top
.
Send a Request to IMDb: Use the requests library to fetch the webpage content.
import requests
from bs4 import BeautifulSoup
url = 'https://www.imdb.com/chart/top'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
Parse the HTML Content: Utilize BeautifulSoup to retrieve the required details.
Handling Pagination: Make sure that your web scraper is able to navigate through pages of IMDb data by detecting and clicking on the links to the next pages.
Error Handling and Robustness: Make sure to include error handling to deal with problems such as network errors, modifications in HTML layout and rate limitations. Utilize try except blocks and record errors, for debugging purposes.
4. Scraping Movie Reviews
Delving into movie reviews can offer a profound understanding of audience perspectives and feelings. Here’s a guide on retrieving reviews, from IMDb.
Identify the Review Pages: Navigate to the movie’s review section. For example, the review page for “The Shawshank Redemption” is https://www.imdb.com/title/tt0111161/reviews
.
Extract Review Data: Scrape the review titles, ratings, and content.
review_url = 'https://www.imdb.com/title/tt0111161/reviews'
review_response = requests.get(review_url)
review_soup = BeautifulSoup(review_response.content, 'html.parser')
reviews = review_soup.find_all('div', class_='text show-more__control')
for review in reviews:
print(review.text)
Analyze Sentiment: Utilize natural language processing (NLP) tools such, as TextBlob or NLTK to assess the feelings conveyed in the reviews. This can offer perspectives on the overall sentiment surrounding a film.
from textblob import TextBlob
for review in reviews:
analysis = TextBlob(review.text)
print(f'Review: {review.text}\nSentiment: {analysis.sentiment}\n')
Handling JavaScript-Rendered Content: Some evaluations could potentially appear dynamically due to the use of JavaScript. You can utilize tools, like Selenium or Puppeteer to interact with content that is rendered through JavaScript.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get(review_url)
reviews = driver.find_elements_by_class_name('text show-more__control')
for review in reviews:
print(review.text)
driver.quit()
Storing Reviews for Analysis: Make sure to save the collected reviews in an organized manner such, as JSON or CSV for examination. This will enable you to conduct processing and analyze sentiments on a broader level.
5. Exporting to JSON and CSV
After you’ve gathered the information, from IMDb you might consider saving it in JSON or CSV format for examination. Exporting the data simplifies sharing studying and presenting it visually.
Export to JSON: JSON is a format, for exchanging data that is both readable and easy to write.
import json
data = {
'movies': [
{'title': 'The Shawshank Redemption', 'year': 1994, 'rating': 9.3},
# More movie data...
]
}
with open('movies.json', 'w') as f:
json.dump(data, f)
Export to CSV: CSV is a file format that is commonly used for storing tabular data.
import csv
data = [
['Title', 'Year', 'Rating'],
['The Shawshank Redemption', 1994, 9.3],
# More movie data...
]
with open('movies.csv', 'w', newline='') as f:
writer = csv.writer(f)
writer.writerows(data)
Using Pandas for Data Export: The Pandas library offers features for manipulating data and is handy, for exporting data as well.
import pandas as pd
df = pd.DataFrame(data[1:], columns=data[0])
df.to_csv('movies.csv', index=False)
df.to_json('movies.json', orient='records', lines=True)
Data Cleaning and Preprocessing: Make sure your data is tidy and organized before you export it. Take care of any missing information delete any duplicates and standardize the formats.
Automating the Scraping and Export Process: Utilize scheduling utilities such as cron for Linux or Task Scheduler for Windows to automate the scraping and exporting procedures. This way you can consistently access up to date information without the need, for involvement.
Conclusion
Mining information from IMDb can provide a plethora of insights, for different needs whether it be personal endeavors or academic investigations. With the help of this guide tailored for 2024 you are equipped with the resources and expertise to extract, evaluate and leverage IMDb data efficiently.
It is crucial to adhere to IMDbs terms of use and handle the data ethically. By adopting the strategies and utilizing the right tools you can leverage the potential of IMDb data to acquire meaningful perspectives and facilitate well informed choices.
Take your data scraping to the next level with IPWAY’s datacenter proxies!