cURL is a tool that works from the command line to move data to and from a server through different protocols like HTTP. One important aspect is how to use cURL with headers in requests. These headers let you share details along with your request, such as authentication details, content types and unique headers.
In this guide we’ll delve into how to use cURL with headers, in depth examples and addressing situations like modifying default headers sending personalized headers submitting empty headers and deleting headers.
Sending HTTP Headers With cURL
When you’re dealing with web services, APIs or web scraping it’s common to include HTTP headers to verify identity define content types handle sessions and share information. cURL offers a method to incorporate these headers in your HTTP requests. In this segment we’ll delve into the basics of sending HTTP headers using cURL touching on both the essentials and complex scenarios.
Understanding HTTP Headers
Prior to delving into the details of utilizing cURL it’s crucial to grasp the significance of HTTP headers. These headers consist of key value pairs transmitted within the request or response message of HTTP transactions. They serve to offer details about the request or response such, as the type of content authentication credentials and personalized metadata.
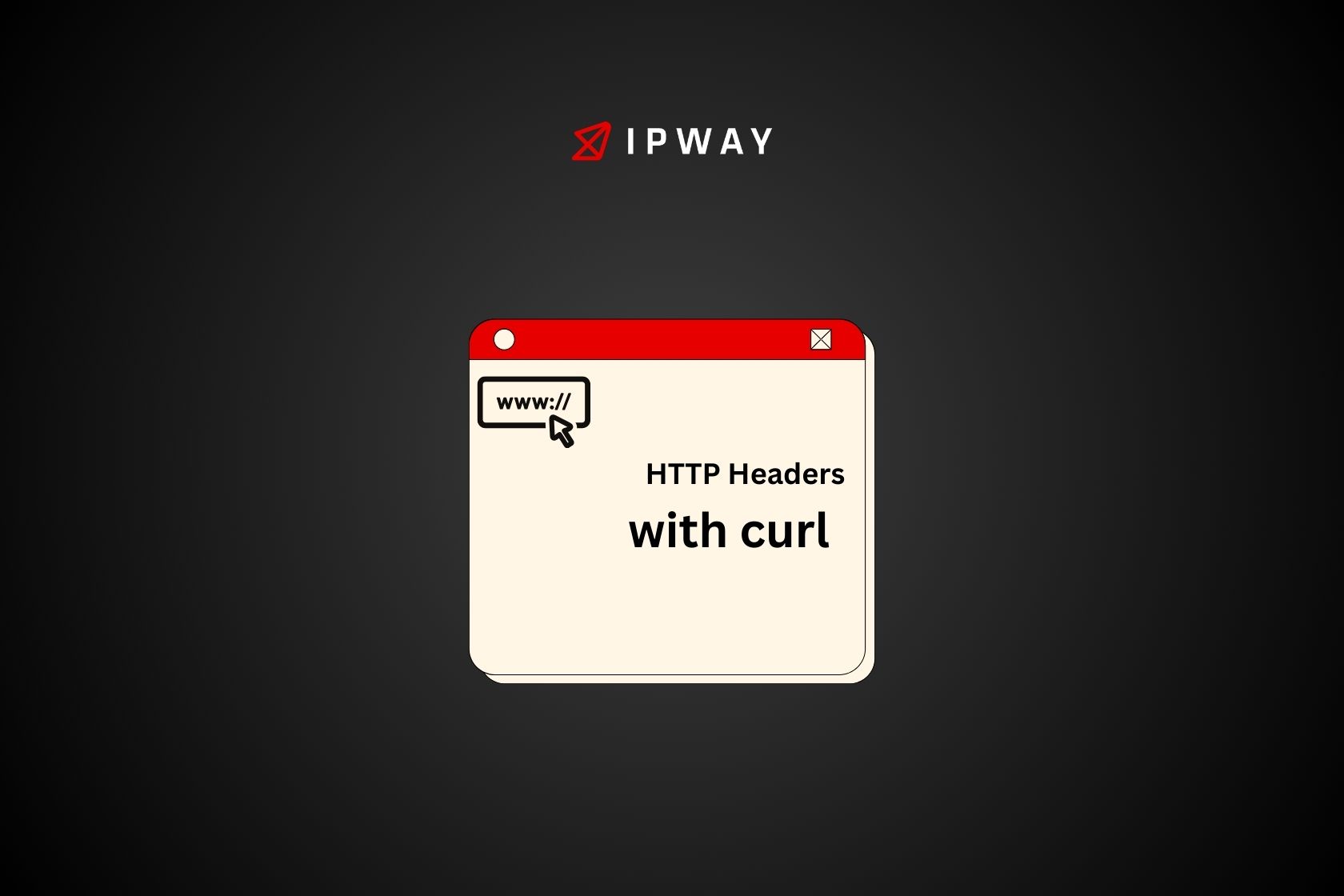
HTTP headers play a critical role in web communication by:
Providing Metadata: Headers provide information that gives context to the request or response aiding servers and clients in interpreting how to manage the data.
Managing Sessions: Headers such as Cookie and Set Cookie are essential for managing sessions, within stateless HTTP transactions.
Specifying Content Types: Headers, like Content Type and Accept show what data format is being sent and what format the client expects in return.
Handling Authentication: Headers like Authorization contain information such as tokens, API keys or user credentials to ensure secure access, to resources.
Importance of HTTP Headers
HTTP headers are vital for web communication as they facilitate the exchange of information, between the client and server influencing the behavior of the web application. Some key uses of HTTP headers include:
Authentication: In web development categories like Authorization serve the purpose of transmitting credentials, such, as API keys or tokens to verify the identity of the user.
Content Negotiation: The Accept and Content Type headers are employed to indicate the types of media that the client can manage and the structure of the information being transmitted.
Session Management: Headers such as Cookie and Set Cookie help handle user sessions. Maintain interactions, between the client and server.
Caching: Headers such as Cache Control and ETag play a role, in handling caching to guarantee that users receive the latest content.
cURL with Headers
HTTP headers, which consist of key value pairs are included in the request or response messages of HTTP transactions to offer details. When using cURL you can define headers by utilizing the flag. Lets delve into the utilization of cURL headers.
How to Use cURL Headers
cURL allows you to specify headers using the -H
or --header
flag. Each header must be specified individually, and multiple headers can be included by repeating the -H
flag.
Basic Syntax for cURL Headers
To include a header in a cURL request, use the following syntax:
curl -H "Header-Name: Header-Value" URL
For example, to set the User-Agent
header:
curl -H "User-Agent: MyCustomUserAgent" https://example.com
This instruction directs a GET request to https;//example.com while including a customized User Agent header.
Sending Multiple Headers
To include headers in one cURL request use the H flag repeatedly for each header:
curl -H "Header1: Value1" -H "Header2: Value2" URL
Example:
curl -H "User-Agent: MyCustomUserAgent" -H "Accept: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://api.example.com/data
This command sends a GET request with three headers:
User-Agent
: Set to “MyCustomUserAgent”
Accept
: Set to “application/json”
Authorization: Set to “Bearer YOUR_ACCESS_TOKEN”
Detailed Examples of Using cURL Headers
Lets take a look, at some real world scenarios and applications where cURL headers play a role.
Example 1: Setting Content-Type and Accept Headers
When you work with APIs it’s typical to define the Content Type of the request body and specify the Accept header to show the intended response format.
curl -H "Content-Type: application/json" -H "Accept: application/json" -d '{"key":"value"}' https://api.example.com/endpoint
In this command:
The Content Type header is defined as “application/json,” which signals that the request body contains JSON data.
The Accept header is configured to request a JSON response, from the server.
The -d
flag sends the JSON payload.
Example 2: Using Authorization Headers
When using APIs that need authentication the Authorization header is employed to transmit credentials, like tokens or API keys.
curl -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://api.example.com/protected-resource
In this command:
The Authorization header is configured with “Bearer YOUR_ACCESS_TOKEN ” to transmit an OAuth2 token, for authentication.
Example 3: Custom Headers for Specialized Use Cases
At times it’s necessary to include personalized headers to transmit application information.
curl -H "X-Custom-Header: CustomValue" https://example.com
In this command:
The X Custom Header is a header configured as “CustomValue,” potentially utilized by the server, for specific operations.
Example 4: Sending Headers with Different HTTP Methods
cURL offers a range of HTTP methods including GET, POST, PUT, DELETE and more. Headers can be utilized with each of these methods.
Sending Headers with a POST Request:
curl -X POST -H "Content-Type: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" -d '{"name":"John Doe"}' https://api.example.com/users
Sending Headers with a PUT Request:
curl -X PUT -H "Content-Type: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" -d '{"name":"John Doe"}' https://api.example.com/users/123
Sending Headers with a DELETE Request:
curl -X DELETE -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://api.example.com/users/123
Default Headers Using the -H Flag
By default when you use cURL it automatically includes headers in its requests, like User Agent.. You have the option to modify these default headers by using the H flag. For example if you want to define an User Agent header you can do so by executing the following command:
curl -H "User-Agent: MyCustomUserAgent" https://example.com
This instruction directs a request to retrieve data from https;//example.com specifying the User Agent header as “MyCustomUserAgent.”
Let’s break down a more complex example where multiple headers are set:
curl -H "User-Agent: MyCustomUserAgent" -H "Accept: application/json" https://api.example.com/data
In this example:
- The
User-Agent
header is set to “MyCustomUserAgent.” - The Accept header is configured to “application/json ” showing that the client is looking for a JSON response.
Sending Custom Headers
Users have the option to create custom headers to include details that are not included in typical HTTP headers. These customized headers prove handy for transmitting information to the server like parameters specific, to an application.
When you want to include a header named X Custom Header in your message you can achieve this by following these steps:
curl -H "X-Custom-Header: CustomValue" https://example.com
This action initiates a GET request, with the X Custom Header configured to “CustomValue.”
Custom headers are frequently utilized in API requests to transmit identifiers, setup choices or additional parameters necessary, for the server. Here is a comprehensive illustration:
curl -H "X-Api-Key: 123456" -H "X-Client-ID: abcdef" https://api.example.com/endpoint
In this example:
The X-Api-Key
header carries an API key used for authentication.
The header X Client ID contains a client identifier that enables the server to monitor the request source.
Sending Empty Headers
Sometimes there are situations where you might have to include a header, with no value. This could be required for API endpoints that need the header to be included but don’t need any particular value assigned to it. To send an empty header in cURL, simply omit the value part after the colon:
curl -H "X-Empty-Header:" https://example.com
When you send this it includes a X Empty Header in the request. Sometimes a blank header is employed in situations where just having the header can cause a particular action on the server without regard, to what it contains.
Remove a Header
Sometimes you may find the need to delete a header that gets added automatically by cURL or a previous command. For instance if you have to eliminate the User Agent header:
curl -H "User-Agent:" https://example.com
When you set the User Agent header to empty in cURL it doesn’t include it in the request. This method comes in handy when you want to make sure that specific headers are not transmitted, especially when handling data or resolving request problems.
Send Multiple Headers
When you’re sending headers in one cURL request it’s simple. Just add H flags to include all the necessary headers. This comes in handy for API interactions that need various metadata components.
curl -H "User-Agent: MyCustomUserAgent" -H "Accept: application/json" -H "X-Custom-Header: CustomValue" https://example.com
This command sends a GET request with three headers:
User-Agent
set to “MyCustomUserAgent”Accept
set to “application/json”X-Custom-Header
set to “CustomValue”
In a more advanced scenario, you might interact with an API that requires authentication, content type specification, and custom application parameters:
curl -H "Authorization: Bearer YOUR_ACCESS_TOKEN" -H "Content-Type: application/json" -H "X-App-Version: 1.0" https://api.example.com/resource
In this example:
- The
Authorization
header is used to pass an OAuth2 token for authentication. - The
Content-Type
header indicates that the request body is in JSON format. - The
X-App-Version
header specifies the version of the client application making the request.
cURL POST With Headers
When using cURL to send headers in a POST request the process is quite similar, to sending them in a GET request. The key difference lies in the fact that POST requests usually involve a request body, which is commonly indicated using the d flag.
For instance, to send a JSON payload along with custom headers:
curl -X POST -H "Content-Type: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" -d '{"key1":"value1","key2":"value2"}' https://api.example.com/resource
In this example:
- The
-X POST
flag specifies that the request method is POST. - The
Content-Type
header indicates that the request body is JSON. - The
Authorization
header carries the access token for authentication. - The
-d
flag sends the JSON payload.
Lets delve into an intricate situation where we engage with a REST API that demands various headers and a JSON payload.
curl -X POST -H "Content-Type: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" -H "X-Request-ID: unique-id-12345" -d '{"name":"John Doe","email":"[email protected]"}' https://api.example.com/users
In this example:
- The
X-Request-ID
header carries a unique identifier for tracking the request. - The
-d
flag sends a JSON payload containing user information.
cURL GET Request With Headers
When using cURL to make a GET request it’s typical to include headers, especially when working with APIs. The GET method is utilized for fetching data from a server and headers can offer information or authentication.
For example, to send a GET request with custom headers:
curl -H "Accept: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://api.example.com/resource
In this example:
- The
Accept
header indicates that the client expects a JSON response. - The
Authorization
header carries the access token for authentication.
A GET request that is more intricate could include headers and query parameters:
curl -H "Accept: application/json" -H "Authorization: Bearer YOUR_ACCESS_TOKEN" "https://api.example.com/resource?param1=value1¶m2=value2"
In this example:
- The URL includes query parameters (
param1
andparam2
) along with their values.
Advanced cURL Techniques With Headers
For users, with some experience and those who are more skilled cURL provides a variety of features to manage situations related to HTTP headers. These methods can boost the versatility and effectiveness of your cURL commands.
Using Config Files for cURL Requests
When you’re making a request make sure to include headers that match the purpose of your request. For instance if you’re calling an API, for JSON data be sure to include the headers indicating that you’re looking for a JSON response.
Content-Type Header:
- Purpose: Specifies the media type of the resource.
- Example: For sending JSON data in a request, you would include
Content-Type: application/json
.
Accept Header:
- Purpose: Informs the server about the types of data the client can process.
- Example: To receive a JSON response from an API, include
Accept: application/json
.
Authorization Header:
- Purpose: Contains the credentials to authenticate a user agent with a server.
- Example: For APIs requiring authentication, you might need to include
Authorization: Bearer <token>
.
User-Agent Header:
- Purpose: Provides information about the user agent originating the request.
- Example:
User-Agent: MyApp/1.0.0 (Windows NT 10.0; Win64; x64)
.
Custom Headers:
- Purpose: Any additional headers required by specific APIs for special purposes.
- Example: Some APIs might require a custom header like
X-Api-Key: <your_api_key>
.
Create a config file (config.txt
) with the following content:
-H "User-Agent: MyCustomUserAgent"
-H "Accept: application/json"
-H "Authorization: Bearer YOUR_ACCESS_TOKEN"
You can then use the -K
flag to tell cURL to use the config file:
curl -K config.txt https://api.example.com/resource
Handling Redirects
By default cURL doesn’t automatically redirect. If you want to follow redirects and include headers, in each redirected request you can use the flag.
curl -L -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://example.com
When using cURL make sure to use the flag for following redirects and remember that the Authorization header needs to be added to every request.
Debugging cURL Requests
When you’re trying to fix issues with cURL requests it’s useful to view all the specifics of the request and response such as headers. Just add the v flag, for output.
curl -v -H "Authorization: Bearer YOUR_ACCESS_TOKEN" https://api.example.com/resource
When using the v flag you can get a breakdown of the request, such, as the headers that were sent and received.
Conclusion
Sending HTTP headers using cURL is crucial for engaging with web services, APIs and web applications. Headers are key, in conveying information, managing request and response behavior and ensuring efficient communication.
In this comprehensive guide, we covered various aspects of sending HTTP headers with cURL, including:
- Changing default headers using the
-H
flag. - Sending custom headers and handling empty headers.
- Removing headers and sending multiple headers.
- Using cURL with POST and GET requests.
- Advanced techniques for handling complex scenarios.
By grasping and utilizing these methods you can boost your skills with cURL. Elevate the potency and adaptability of your online engagements. Whether you’re engaged in API connections data extraction, from websites or other internet related activities mastering the management of HTTP headers will prove to be an asset.
Discover how IPWAY’s innovative solutions can revolutionize your experience for a better and more efficient approach.