When it comes to creating software being efficient is crucial. Whether you’re building a website working on AI algorithms, or improving processor speed, how fast and responsive your code is can greatly affect how users experience your system. Using Python cache is a method to boost the efficiency. In this article we will discuss about Python cache techniques, various caching approaches and show you how to apply them to speed up your code.
What is a Cache in Programming?
In programming a cache is like a kind of short term memory that stores information or results from calculations that are time consuming to get or process. The main goal of using a cache is to make data retrieval and lighten the workload on systems by keeping often used or complex data in an easier to reach place, such as in the computers memory (RAM) instead of redoing the calculations or fetching it again from a slower farther source such, as a database or an external API.
The idea behind caching is based on the concept of locality which suggests that software often accesses the data or instructions repeatedly. Caching takes advantage of this pattern by keeping accessed data nearby reducing the time required to retrieve it for future use.
Simply put, when a program needs to access data it first checks the cache. If the data is already in the cache (referred to as a cache hit) the program can quickly retrieve it. However if the data is not in the cache (a cache miss) then the program has to go through a process of fetching the data from its original source before storing it in the cache, for potential future access.
Caching is a ubiquitous concept across many areas of computing:
Web Browsers: Web browsers utilize caching to save duplicates of web pages, images and additional resources directly on a users device. This enables the browser to speed up page loading when a user returns to a website by fetching data, from the cache of re downloading it from the internet.
Databases: Many database systems use caching techniques to save outcomes of queries or commonly accessed information. This helps lessen the strain on the database and quickens response times, for recurring queries.
Operating Systems: Operating systems utilize caching to enhance the speed of accessing disks by storing accessed disk blocks in memory. This practice, referred to as disk caching plays a role, in boosting the efficiency of file system tasks.
Content Delivery Networks (CDNs): Content delivery networks utilize caching to save duplicates of content in different global locations. Whenever a user asks for content the CDN delivers it from the cache decreasing delays and enhancing loading speeds.
Central Processing Units (CPUs): In the realm of computer architecture processors utilize tiers of caching, such as L1, L2 and occasionally L3 caches to retain duplicates of commonly used instructions or data in proximity, to the CPU. This practice aims to reduce the retrieval time required from the memory (RAM).
The success of a cache relies on its hit rate, which shows the percentage of requests that can be fulfilled from the cache of the primary source. A hit rate suggests a better performing cache resulting in quicker program operation and less strain, on underlying assets.
In the realm of Python programming caching is frequently utilized at tiers ranging from basic in memory caching to sophisticated distributed caching setups tailored to suit the requirements of the application. Python provides pre built utilities and frameworks for enabling Python cache, simplifying the process for developers to fine tune their applications and realize substantial performance improvements.
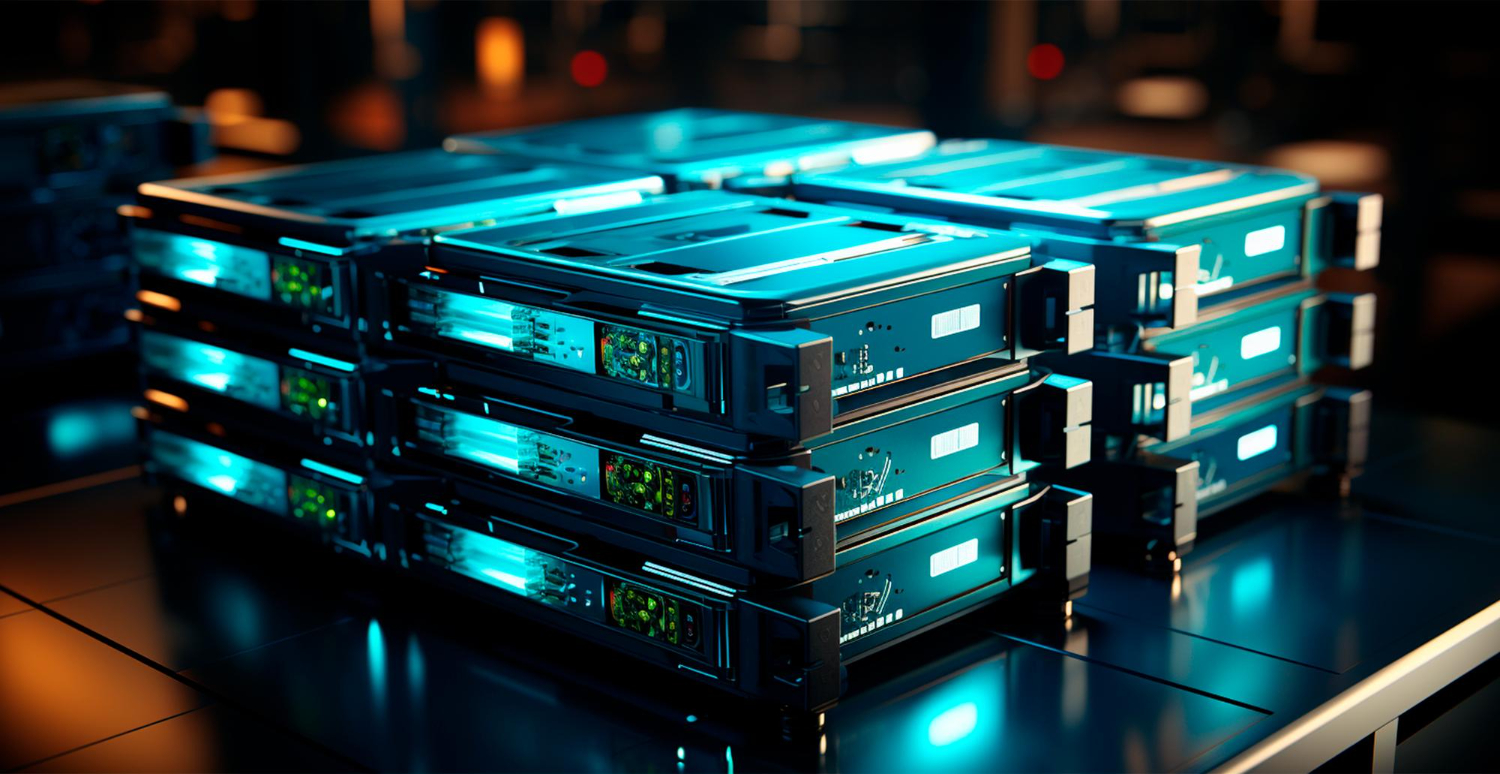
The Purpose of Caching
In software development caching plays a role for developers to make informed choices on when and where to apply caching in their apps.
Reduced Access Time
One major advantage of caching is the faster access time it provides. By storing data or computational outcomes in a cache future requests for that information can be fulfilled from the cache of the primary source. This results in a decrease in the time required to retrieve the data particularly when the original source is sluggish or costly to access. For example in a web application fetching data from a cache proves to be speedier, than querying a database each time a user initiates a request.
Reduced System Load
Using caching can also lessen the burden on systems. Of carrying out complex calculations or database searches every time the system can respond to requests from the cache. This approach allows the system to manage a volume of requests using existing resources. This becomes especially crucial in settings as easing the strain, on the backend can result in systems that are more adaptable and robust.
Improved User Experience
From the viewpoint of a user caching results in response times and a more seamless user experience. Whether it involves loading a webpage or handling a request in a desktop application users anticipate feedback. Caching enables developers to fulfill these expectations by offering access, to regularly requested information.
Common Use Cases for Caching
Caching is a resource that can be utilized in different situations. Below are some scenarios where caching is highly beneficial:
Web Applications
In the realm of web development caching is frequently employed to retain the outcomes of costly database inquiries or API requests. Storing these outcomes allows for responses to follow up requests thus boosting the applications efficiency. To illustrate a news platform could store the outcomes of its sought after articles in cache lessening the burden, on the database and hastening page loading times for users.
from functools import lru_cache
@lru_cache(maxsize=100)
def fetch_popular_articles():
# Simulate a time-consuming database query
time.sleep(2)
return ["Article 1", "Article 2", "Article 3"]
# First call will take time
print(fetch_popular_articles())
# Subsequent calls will be fast
print(fetch_popular_articles())
Machine Learning
In the field of machine learning caching is a technique that saves the outcomes of calculations, like model predictions or preprocessing outputs. By storing these outcomes developers can cut down on training and inference time enabling them to make progress in refining their models.
from functools import lru_cache
@lru_cache(maxsize=None)
def preprocess_data(data):
# Simulate a time-consuming preprocessing step
time.sleep(5)
return data.lower()
data = "SOME LARGE TEXT DATASET"
processed_data = preprocess_data(data)
Central Processing Units (CPUs)
Storing used instructions or data near the processor is a crucial aspect of CPU design. This helps speed up program execution by reducing the time needed for the CPU to retrieve information, from the memory.
Caching Strategies
Developers have a range of caching strategies at their disposal, in Python cache each offering benefits and applications. Among the used strategies are:
- Memoization: This includes storing the outcomes of function calls according to their inputs. When the function is called again with the inputs it retrieves the stored result instead of recalculating it. In Python you can easily apply this by using the lru_cache decorator found in the functools module.
- Time-based Caching: This approach includes storing data for a period. Once the allotted time elapses the cache becomes outdated prompting the need to retrieve or recalculate the data. This method proves beneficial when dealing with changing data and aiming to keep the cache synchronized with updates.
- Cache Invalidation: To keep the cached data up to date and relevant it’s important to eliminate any obsolete information from the cache. This can be done manually like when a certain event triggers it or automatically based on a time to live (TTL) setting. Ensuring cache invalidation is key, to preserving the correctness and timeliness of stored data.
How to Implement Python Cache
Python offers tools and libraries for incorporating caching. A popular method is using the functools.lru_cache decorator making it simple for developers to memoize functions. In the following discussion we’ll delve into how to set up Python cache by utilizing this method along with techniques.
Using functools.lru_cache
The functools.lru_cache decorator, in Python is a tool that automatically stores the outcomes of a function depending on its input arguments. It employs a strategy known as Recently Used (LRU) caching, where the oldest accessed items are removed once the cache is full.
Here’s an example of how to use lru_cache
:
from functools import lru_cache
@lru_cache(maxsize=32)
def compute_factorial(n):
if n == 0:
return 1
else:
return n * compute_factorial(n-1)
# The first call will compute and cache the result
print(compute_factorial(5)) # Output: 120
# Subsequent calls with the same argument will use the cache
print(compute_factorial(5)) # Output: 120 (from cache)
In this instance the compute_factorial function computes the factorial of a number. The outcomes are stored in a cache so when the function is invoked with the input again it retrieves the stored result thereby conserving computing time.
Custom Caching Mechanism
To have influence, on how caching works you can create your own custom caching system. This method comes in handy when you want to store objects or when the caching strategy must be customized for particular scenarios.
class SimpleCache:
def __init__(self):
self.cache = {}
def get(self, key):
return self.cache.get(key)
def set(self, key, value):
self.cache[key] = value
def invalidate(self, key):
if key in self.cache:
del self.cache[key]
# Example usage
cache = SimpleCache()
cache.set("user_123", {"name": "Alice", "age": 30})
# Retrieve cached data
print(cache.get("user_123"))
# Invalidate cache
cache.invalidate("user_123")
print(cache.get("user_123"))
In this instance the SimpleCache class presents functions, for establishing, retrieving and nullifying cached information. While this method allows for adaptability it demands attention to uphold cache coherence and elimination.
Conclusion
Python cache can greatly boost the speed of your programs. Whether you’re building a website using machine learning models or optimizing CPU tasks knowing how to use caching can make your code run faster and more efficiently. By using Python cache built in tools, like functools.lru_cache or creating your caching methods you can speed up data access lessen system strain and enhance user interactions.
Adding Python cache to your projects entails thinking about the particular scenario and the type of data being stored. When implemented properly caching can significantly enhance your ability to create scalable and efficient applications.
Make sure you keep an eye on handling cache invalidation and monitoring the size of the cache to avoid problems, like outdated data or excessive memory usage. By implementing these tactics you’re making strides in becoming proficient with Python cache.
Boost your caching in Python effortlessly with IPWAY’s reliable proxies. Check out IPWAY today for top-notch security and performance!