Web scraping has become a tool for individuals passionate about data, online marketers and software developers, aiming to gather valuable insights from search engines.
While Google usually takes the spotlight, Bing is also worth considering for those in broadening their data pool. This article delves into the process of extracting Bing search results using Python, addressing various aspects from initial setup to overcoming obstacles inherent in scraping search engines.
Is allowed Scraping Bing?
Before delving into the specifics, it’s important to touch upon the moral aspects of extracting information from Bing. Similar to search engines, Bing’s terms of service forbid unauthorized data extraction mainly to safeguard their information and uphold server stability. Nevertheless, scraping Bing for research reasons while following ethical standards is usually deemed appropriate.
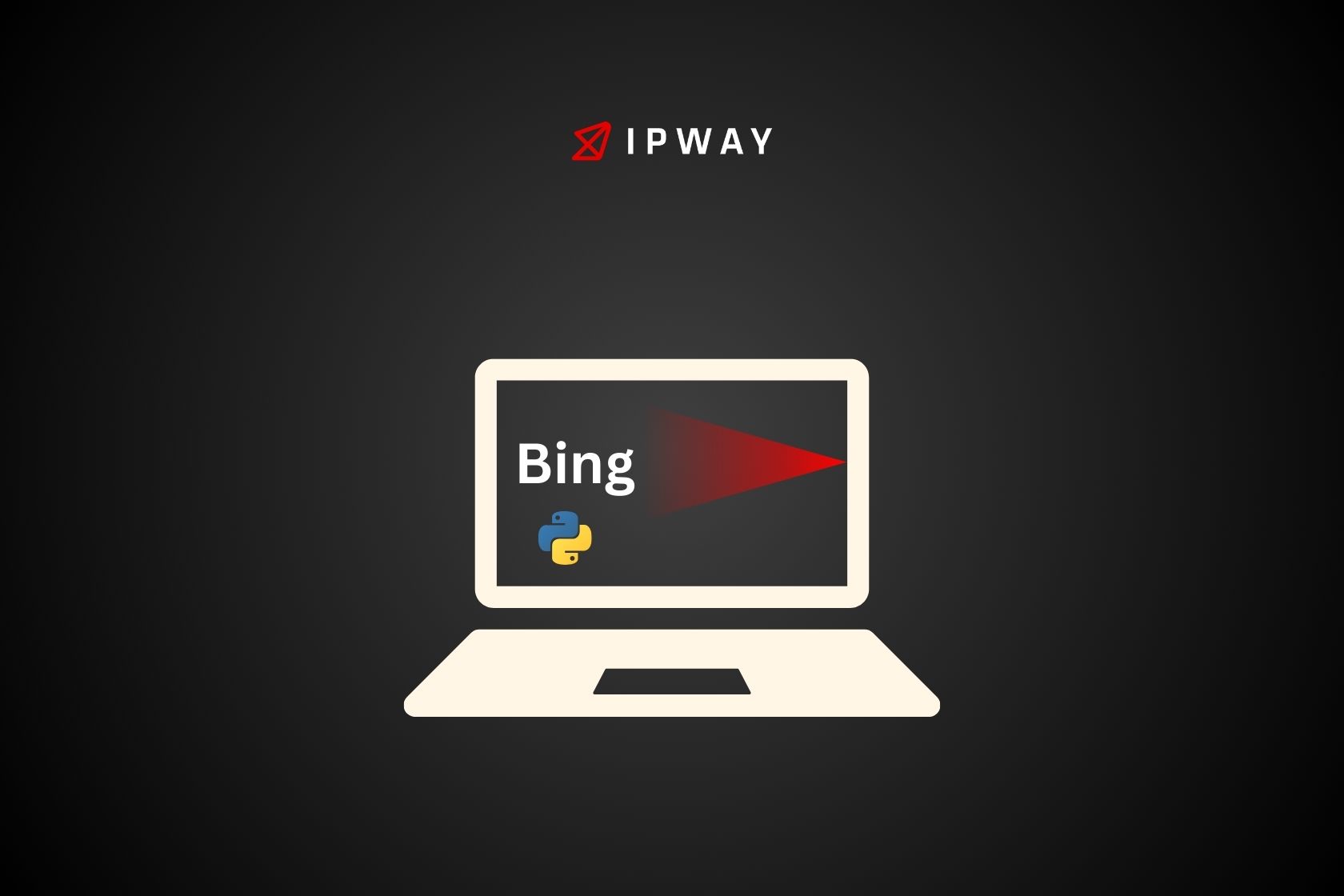
To mitigate the risk of being blocked, it’s advisable to:
- Make sure to space out your requests so that you don’t put much strain on Bings servers.
- Switch up your IP address frequently with the help of a proxy service.
- Remember to honor Bing’s robots.txt file, which specifies the sections of the website that automated bots, should not access.
Difficulties with Scraping Bing
Scraping data, from Bing presents difficulties primarily because of the search engines efforts to prevent scraping. These efforts may involve:
Rate limiting: Bing keeps an eye on how requests are made and might restrict access, for IPs that send too many requests in a short timeframe.
CAPTCHAs: Bing might show CAPTCHA tests to confirm if the user is a human, especially when suspicious behavior is noticed.
Dynamic content: Bings search results can be difficult to extract using scraping techniques because they are frequently loaded dynamically with JavaScript.
Overcoming these obstacles requires a planned strategy to guarantee the success and continuity of your web scraping efforts.
Setting Up the Project Environment
Before diving, into Bing scraping it’s important to establish our Python environment. Here’s a detailed guide to help you kick things off:
Install Python and Pip:
Make sure you’ve got Python set up on your computer. You can get it from python.org. Pip, which is Pythons package installer usually comes with recent Python setups.
Create a Virtual Environment:
Creating an environment is a smart approach to organizing the dependencies that are unique, to your project.
python -m venv bing_scraper_env
source bing_scraper_env/bin/activate # On Windows use `bing_scraper_env\Scripts\activate`
Install Required Libraries:
We will utilize requests, for sending HTTP requests BeautifulSoup to parse HTML and lxml for parsing.
pip install requests beautifulsoup4 lxml
Proxy Setup (Optional but Recommended):
To prevent getting banned and enhance the effectiveness of your data scraping its recommended to utilize a proxy service. IPWAY provides proxy solutions that enable you to switch IPs and stay anonymous.
pip install requests[socks] # If using SOCKS proxies
SERP Scraper API Query Parameters
When analyzing Bings Search Engine Results Page (SERP) through web scraping it is important to grasp the setup of the query parameters. Bings search links commonly include parameters, like:
q
: The search query string.count
: The number of results to return per page.offset
: The index of the first result to return, useful for pagination.mkt
: The market or region setting for the search, e.g.,en-US
for the United States.
Here’s an example of a Bing search URL:
https://www.bing.com/search?q=python+web+scraping&count=10&offset=0&mkt=en-US
In this URL:
q=python+web+scraping
: Searches for the keyword “python web scraping.”count=10
: Returns 10 results per page.offset=0
: Starts from the first result.mkt=en-US
: Sets the search market to the United States.
Knowing these factors will help you adjust your web scraping process to gather information more effectively.
Scraping Bing SERPs for Any Chosen Keyword
Now that we have arranged our workspace and grasped the query details lets explore the procedure of extracting information, from Bing search engine results pages.
Step 1: Crafting the URL
Begin by creating the search link using the keyword you wish to gather information on. For example if you’re looking to retrieve data, on “searching bing”:
import requests
from bs4 import BeautifulSoup
# Define the search query
query = "scraping bing"
url = f"https://www.bing.com/search?q={query.replace(' ', '+')}&count=10"
print(f"Scraping URL: {url}")
Step 2: Sending the HTTP Request
Lets proceed by sending a HTTP GET request to Bing, with the help of the requests library.
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36"
}
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Request successful!")
else:
print(f"Failed to retrieve results: {response.status_code}")
It is crucial to include an User Agent string, in the headers to prevent being identified as a bot.
Step 3: Parsing the HTML Content
After obtaining the HTML content you can utilize BeautifulSoup to extract the search results.
soup = BeautifulSoup(response.text, 'lxml')
# Extracting search result titles and links
results = []
for result in soup.find_all('li', class_='b_algo'):
title = result.find('h2').text
link = result.find('a')['href']
results.append({"title": title, "link": link})
# Display the results
for index, result in enumerate(results):
print(f"{index + 1}: {result['title']} - {result['link']}")
This piece of code pulls out the title. Link for every search result, on the initial page. You can adjust it to retrieve information like excerpts or dates.
Step 4: Handling Pagination
To gather information from search result pages you will have to modify the offset parameter in the website address and navigate through each page sequentially.
def scrape_bing(query, pages=1):
results = []
for page in range(pages):
offset = page * 10
url = f"https://www.bing.com/search?q={query.replace(' ', '+')}&count=10&offset={offset}"
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'lxml')
for result in soup.find_all('li', class_='b_algo'):
title = result.find('h2').text
link = result.find('a')['href']
results.append({"title": title, "link": link})
return results
# Example usage:
all_results = scrape_bing("scraping bing", pages=5)
for index, result in enumerate(all_results):
print(f"{index + 1}: {result['title']} - {result['link']}")
You can use this feature to extract data from pages by indicating the number of pages you wish to extract from.
Conclusion
Using Python to extract search results from Bing is a way to gather information whether its for analyzing SEO strategies conducting competitive research or aggregating content. However it’s crucial to handle web scraping in order to avoid triggering Bings anti scraping measures.
To improve the effectiveness of your scraping activities and reduce the chances of getting blocked consider utilizing a proxy service such as IPWAY. With IPWAY you can rotate your IP addresses. Maintain the necessary anonymity for successful scraping on Bing, without any disruptions. Their proxy solutions are specifically designed for web scraping purposes ensuring that you can collect the data you require without encountering rate limits or CAPTCHAs.
Are you looking to scale your web scraping projects and avoid IP blocks? IPWAY offers top-tier proxy solutions that can help you scrape Bing and other search engines with ease.