Web Scraping C#: In todays technology driven era web scraping plays a role in making informed decisions based on data. C# stands out as an option for developers interested in creating web scraping solutions due to its solid framework and extensive library support.
This manual offers an overview of the steps involved in web scraping C# including choosing appropriate libraries efficiently handling and leveraging scraped data and tackling issues such, as data protection and potential IP restrictions.
Top Web Scraping C# Libraries
Adopting the right tools can streamline web scraping. Explore the top NuGet scraping libraries for C#:
HtmlAgilityPack is known for being the top notch C# scraping tool. It allows users to easily fetch web pages analyze their HTML content pick out HTML elements and retrieve data seamlessly.
The HttpClient stands out as the premier C# HTTP client, highly valued for its adaptability, in handling web scraping duties. It streamlines the process of sending HTTP requests providing efficient asynchronous capabilities.
Selenium WebDriver, compatible with multiple programming languages, enables the creation of automated tests for web applications and can be utilized for web scraping tasks as well.
Puppeteer Sharp, the C# adaptation of Puppeteer, offers headless browser functionality and facilitates scraping of dynamic content pages.
Building a Web Scrape with C#
Developing a web scraper with C# follows an approach that includes configuring your development setup coding to send HTTP requests extracting data, from the obtained HTML and organizing the output information efficiently. Here’s a step-by-step breakdown to guide you through the development of a robust C# web scraper:
Setup and Environment Preparation
Create a new C# Project: Begin by creating a console application, in Visual Studio or your chosen IDE that works with C#. Name your project based on the purpose of your web scraping task.
Install Necessary Packages: To get started you can install the required libraries using the NuGet Package Manager. When it comes to scraping data HtmlAgilityPack is quite handy for simplifying HTML parsing. If you have complex requirements such, as CSS selector support you might want to look into AngleSharp.
Configure the Project Settings: Make sure that your project is aimed at the edition of the.NET framework or.NET Core to guarantee that it works well with the libraries and APIs you plan to utilize.
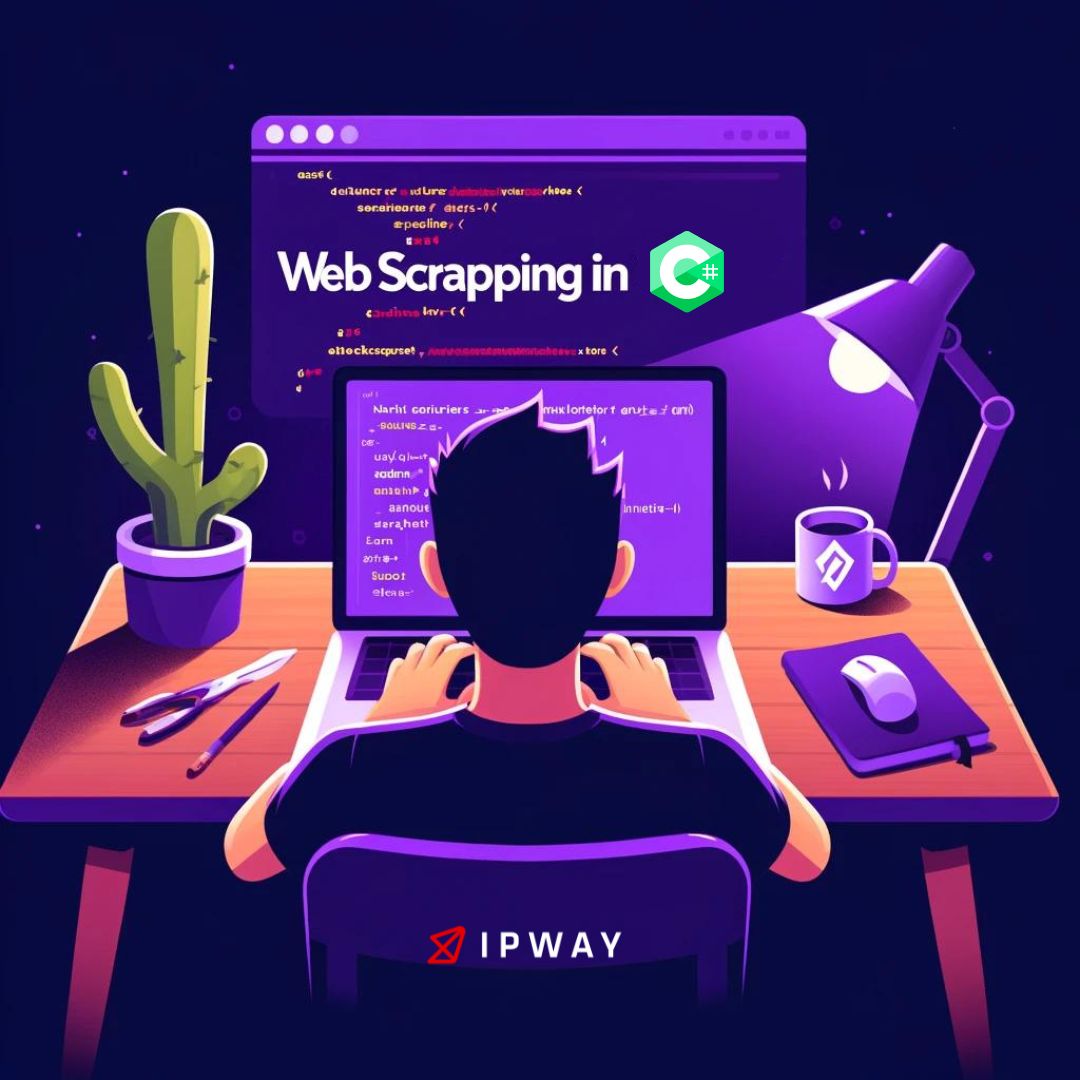
Fetching HTML Content
HttpClient Usage: To send requests to the web create an HttpClient object. It’s better to use HttpClient of WebClient because you can use it for many requests making it more efficient for tasks, like web scraping C#.
Handling Web Requests: Set up the headers in your HTTP requests to imitate a web browser, which helps lower the risk of getting blocked by the website. This involves adjusting the user agent accept and other essential headers.
Asynchronous Requests: To boost your scrapers efficiency consider sending requests. This approach becomes especially handy when expanding the scrapers capabilities to process URLs.
HttpClient client = new HttpClient();
client.DefaultRequestHeaders.Add("User-Agent", "Mozilla/5.0 (Windows NT 10.0; Win64; x64)");
string html = await client.GetStringAsync("http://example.com");
Parsing the HTML
Load HTML into Parser: You can utilize HtmlAgilityPack or AngleSharp to access the HTML content you fetched. These tools offer parsing features to handle imperfect HTML by correcting typical mistakes, which enhances the web scraping C# processs reliability.
Extracting Data: Utilize XPath or CSS selectors to navigate the HTML document. Retrieve the necessary information. Both HtmlAgilityPack and AngleSharp provide support, for these selectors although their syntax and functionalities might differ.
HtmlDocument document = new HtmlDocument();
document.LoadHtml(html);
HtmlNodeCollection nodes = document.DocumentNode.SelectNodes("//div[@class='product']");
foreach (var node in nodes)
{
Console.WriteLine(node.InnerText.Trim());
}
Data Storage and Management
Storing Extracted Data: You might decide to save the extracted information for your project in ways, such as a database, a CSV file or any other storage method. When it comes to databases you could think about using Entity Framework for incorporation, with C# programs.
Error Handling: Make sure to set up error handling to deal with problems such as network outages, parsing issues or modifications, in the websites HTML layout. Keeping a record of these errors will assist in diagnosing and upkeeping the scraper.
Optimization and Scalability
Multithreading and Task Parallelism: To improve the efficiency of your web scraper especially when expanding to process pages or websites consider incorporating multithreading or utilizing asynchronous methods to execute tasks simultaneously.
Rate Limiting: To avoid putting much strain on the server you’re targeting and to lower the chances of getting banned make sure to set up rate limits and follow the guidelines outlined in the sites robots.txt file.
Scraping Static Content Websites in C#
When you scrape content websites you’re essentially pulling information from web pages that don’t need client side scripting to show their content. These sites usually consist of HTML and CSS which makes them easier to extract data from using C#. Here are some thorough guidelines and factors to keep in mind when scraping content, with C#.
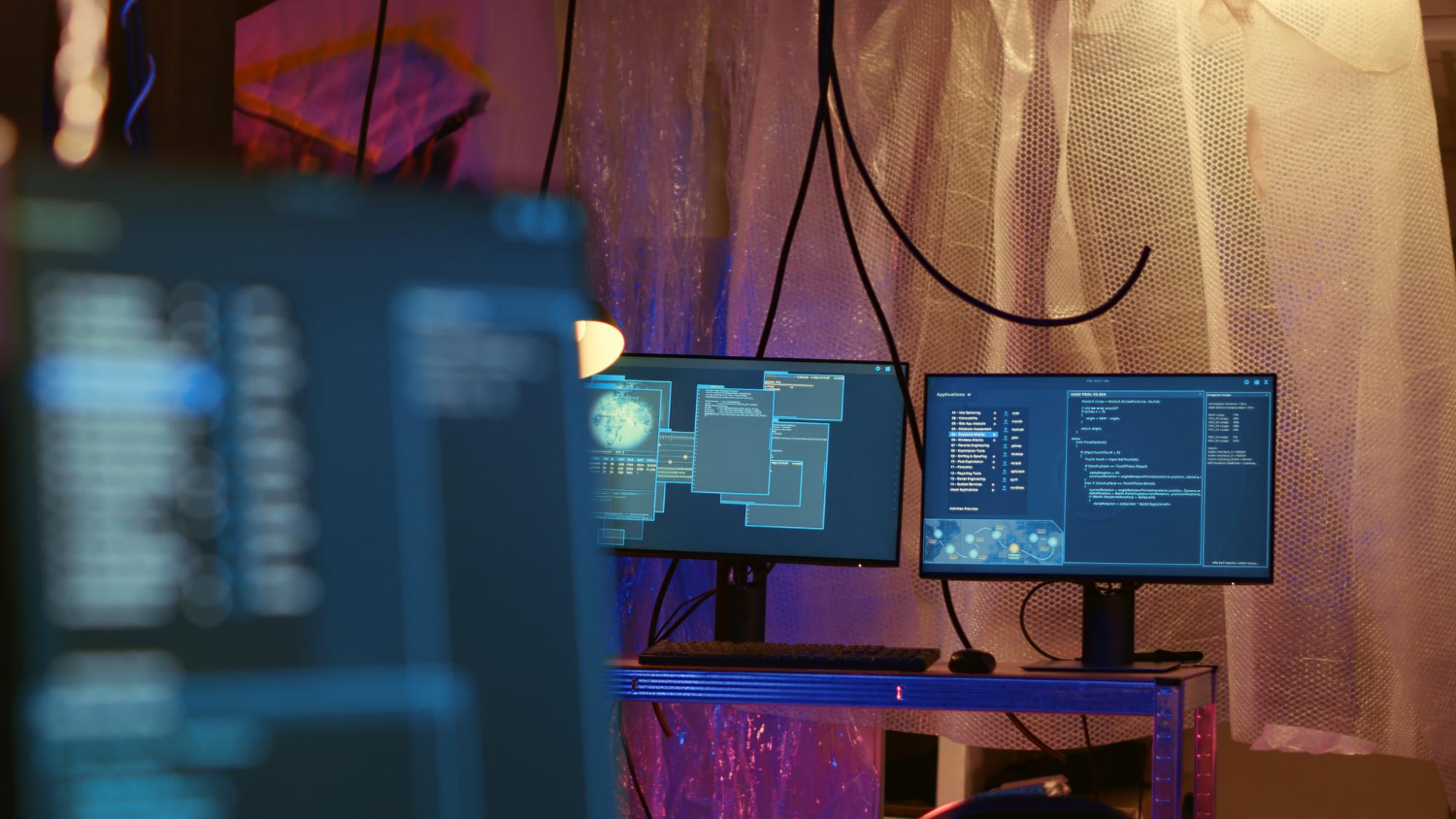
Understanding Static Websites
Nature of Static Sites: Static websites provide content to all users. In contrast to websites they do not depend on client side scripting such, as JavaScript for displaying content resulting in all information being included directly in the HTML provided by the server.
Identifying Data Structure: Prior to starting the scraping process it’s important to examine the HTML layout of the webpage to locate where the necessary data is saved. Utilize resources such as Chrome Developer Tools to aid in this examination enabling you to navigate through the DOM and experiment with XPath or CSS selectors, within the browser.
Preparation for Scraping
Setting Up the Environment: When starting a C# project begin by setting up your development environment. Create a console application in Visual Studio and make sure to install required libraries such as HtmlAgilityPack, for parsing HTML.
Develop a Fetching Strategy: Figure out the method, for getting onto the website. In case the website contains an amount of pages you might have to create URLs automatically or go through them page by page using a loop.
Implementing the Scraper
HTTP Request Handling: Utilize HttpClient for sending requests, to the website. Be sure to handle headers to imitate a browser request decreasing the chances of getting blocked by the server.
HTML Parsing:
- Loading HTML: After you get the HTML content you can load it into an HtmlDocument object using HtmlAgilityPack. This object is, like a map of the HTML that helps you move around and find things in the structure.
- Data Extraction: Utilize XPath or CSS selectors to. Extract information. For instance when scraping product information identify the selectors that can gather details, like product names, prices, descriptions and more.
using HtmlAgilityPack;
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class StaticContentScraper
{
public async Task ExtractContent(string url)
{
HttpClient client = new HttpClient();
var response = await client.GetAsync(url);
var pageContent = await response.Content.ReadAsStringAsync();
HtmlDocument doc = new HtmlDocument();
doc.LoadHtml(pageContent);
var nodes = doc.DocumentNode.SelectNodes("//div[contains(@class, 'product-info')]/h1");
foreach (var node in nodes)
{
Console.WriteLine("Product Name: " + node.InnerText.Trim());
}
}
}
Handling Common Challenges
Rate Limiting and Ethics: To prevent overloading the server it’s advisable to insert time gaps between requests, especially when interacting with non commercial websites.
Error Handling: It’s important to have error management in place. Be sure to address issues with network connectivity handle any HTTP errors that may arise and adapt smoothly to any changes, in the HTML structure. Make sure to keep a record of these occurrences for review and fine tuning of your web scraping tool.
Testing and Maintenance
Regular Testing: Make sure to check your web scraper to confirm that it is still working properly especially if you are using it for ongoing data gathering.
Maintenance: Remember to update your code and software libraries. Stay vigilant by checking the website for any alterations, in its layout or rules related to web scraping C#.
What To Do With the Scraped Data
After gathering information from a website it is important to handle it in a responsible and ethical manner. This includes taking into account aspects such as privacy following regulations and implementing technical methods to maintain consistent access to the data sources. Here is a comprehensive guide, on how to manage the data you have gathered through scraping:
Data Privacy With Proxies
Understanding Proxies: Proxies act as intermediaries that link your web scraping tool to the target website while concealing your servers IP address. Using proxies is essential for maintaining anonymity, which’s important, for protecting user privacy and adhering to data protection laws.
Implementing Proxy Rotation: To make use of proxies set up a rotation system that utilizes a group of proxies and switches, between them. This method not enhances privacy but also lowers the chances of a single proxy being compromised or flagged.
Avoid IP Banning
Rate Limiting: Make sure to limit the rate of your scraping activity to avoid sending a number of requests within a brief timeframe as this could result in getting banned. Following the guidelines outlined in the websites robots.txt file and terms of service is a recommended approach that shows respect, for the websites rules.
User-Agent Rotation: Rotating user agent strings in addition to changing IP addresses can simulate the actions of browsers and devices making it less likely for detection, as a scraper.
Rotating IP Addresses
IP Rotation Strategy: Consider utilizing an IP rotation service or configuring a proxy server that permits regular IP alterations. This approach aids in evading detection and evenly spreads the workload, among servers lessening the risk of overwhelming any individual server.
Regional Scraping
Compliance with Local Laws: When collecting information from areas it’s important to adhere to the data privacy laws specific to each region like GDPR in Europe CCPA in California and other applicable regulations. This could mean storing data on servers, within the region protecting information through anonymization and obtaining consent before gathering any data.
Localized Scraping Techniques: To lower the chances of getting blocked and to accurately capture differences use IP addresses from the specific area. This is crucial, for platforms that tailor content according to user location.
Implementing These Strategies
To effectively put these tactics into action think about incorporating software tools that handle rotation and automatic IP changes. Also creating alerts, for system behaviors that could signal blocks or access problems can aid in keeping your scraping activities running smoothly.
Conclusion
Utilizing web scraping C# proves to be an asset for developers especially in tasks like data analysis, market research and automation. With the libraries and following recommended methods you can effectively collect essential data while managing the intricate aspects of web scraping C#, including legal concerns and technical obstacles such, as IP restrictions and safeguarding data privacy.
This detailed manual is designed to provide you with the information needed to carry out web scraping C# effectively. Keep in mind that successful scraping involves more, than collecting data; it also requires a responsible and ethical approach.
Discover how IPWAY’s innovative solutions can revolutionize your web scraping experience for a better and more efficient approach.