Ever felt like you’re on the brink of discovering something groundbreaking, only to be held back by the sheer volume of data sprawled across the web? Enter the realm of web scraping Python—a magician’s wand for data enthusiasts and professionals alike. This guide doesn’t just scratch the surface; it dives deep, offering you a step-by-step tutorial on extracting web data with precision and efficiency.
Whether you’re a beginner looking to get your hands dirty or a seasoned pro aiming to refine your skills, you’re in the right place. Let’s unravel the secrets of web scraping with Python, transforming complexity into simplicity.
- What Is Web Scraping Python?
- Building a Web Scraper: Python Prepwork
- Getting to the Libraries
- WebDrivers and Browsers
- Importing and Using Libraries
- Picking a URL
- Defining Object and Building Lists
- Extracting Data With a Python Web Scraper
- Exporting the data to CSV
- Exporting the data to Excel
- Web Scraping Python – Best Practices
- Conclusion
What Is Web Scraping Python?
Web scraping, fundamentally involves collecting data from the internet using programming techniques. This could include tasks like retrieving prices of products aggregating articles or creating contact information databases.
Python is widely regarded as a tool for handling these activities due to its user friendly nature and extensive library support. The focus isn’t on extracting data; it’s also, about executing this process effectively while adhering to the unspoken guidelines of the web.
Building a Web Scraper: Python Prepwork
When you start web scraping with Python the first thing you need to do is get your environment ready. This includes making sure that Python is installed on your computer. With the new features and enhancements in the latest versions its recommended to opt for Python 3.x. This setup acts as the foundation getting your system ready, for the tasks and obstacles you’ll encounter in web scraping.
After setting up it’s important to get to know the Python libraries that’re key for web scraping. Tools like BeautifulSoup and Scrapy play a role in a web scrapers toolkit. BeautifulSoup is well known for its user approach making it a great choice for beginners. On the hand Scrapy is suited for more advanced scraping tasks providing a solid framework, for scraping projects. This initial phase involves choosing the tools and grasping their capabilities, which will greatly impact the efficiency and success of your web scraping ventures.
Getting to the Libraries
Exploring further into the realm of web scraping using Python you soon understand the significance of selecting the libraries. These libraries serve as more, than tools; they act as your guides through the complex structure of HTML and JavaScript that underpins contemporary websites. In this domain there are two players. BeautifulSoup and Scrapy. Each offering distinct advantages suited to various facets of web scraping activities.
BeautifulSoup is known for its user interface, which makes it a great option for beginners entering the realm of web scraping. It simplifies the process of parsing HTML documents allowing users to navigate search and make changes to the parse tree with coding. Despite its simplicity BeautifulSoup doesn’t compromise on effectiveness. It proves to be a tool for projects that demand fast results and easy data extraction, from uncomplicated websites.
Scrapy on the hand provides a robust framework tailored for large scale web scraping tasks. With features that include handling link navigation and managing requests seamlessly Scrapy stands out as the preferred option, for building sophisticated web crawlers required to navigate through numerous pages or entire websites efficiently. Its design enables the development of adaptable scraping guidelines catering to projects that require intricate and detailed processes.
If you want to enhance your web scraping abilities you can consider using tools such as Selenium. This becomes particularly useful when dealing with websites that heavily rely on JavaScript and require user interactions, such as clicking buttons or filling out forms. Selenium simulates these interactions allowing you to extract data that may not be accessible, through the HTML of the webpage.
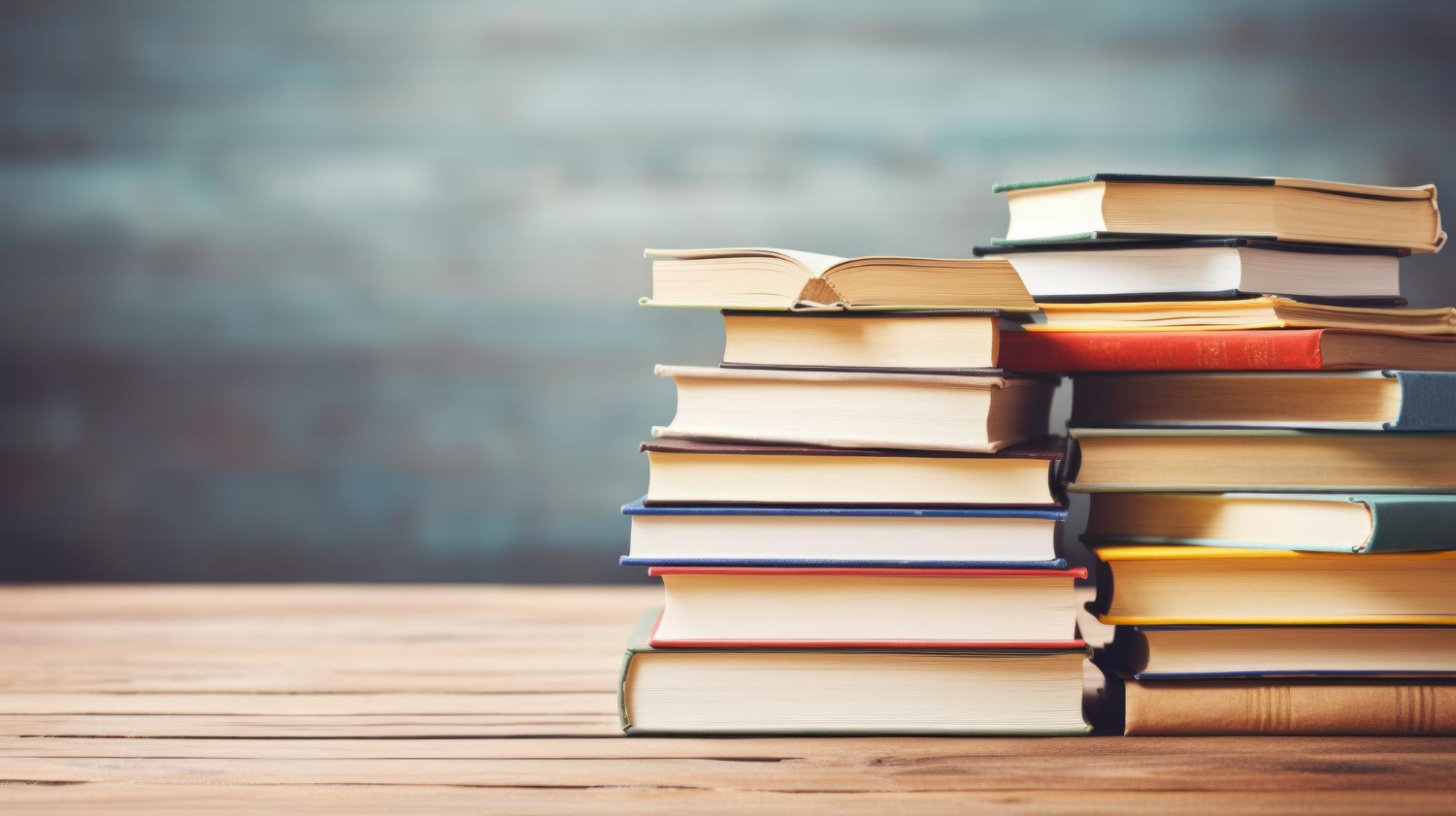
WebDrivers and Browsers
When starting a web scraping project with Python it’s essential to understand the role played by your script in interacting with web pages. This is where WebDrivers and browsers step in acting as the link that connects your code to the changing content of the internet. WebDrivers essentially serve as drivers for browsers allowing automated control, over web browsers so that your script can carry out tasks just like a real person navigating through the website. This functionality is particularly important when scraping websites where content might be loaded asynchronously using JavaScript or when user input is needed to access the desired information.
Selenium WebDriver is quite impressive in this field providing a range of tools to help with automating tasks for web applications. Using Selenium allows you to control a browser visit web pages click on links fill in forms and manage pop ups through code. It works well with browsers such as Chrome (using ChromeDriver) Firefox (with GeckoDriver) and Safari, among others. This adaptability ensures that your automation tool can interact with websites like a human would making it possible to access content that may not be easily reachable, through basic HTML analysis alone.
Incorporating Selenium WebDriver into your web scraping process entails configuring the browser driver and specifying the browser you want to automate. For example when automating Google Chrome, with ChromeDriver you need to download the corresponding ChromeDriver that matches your Chrome version and set up your script to utilize this driver for launching and managing the browser. This setup enhances the capabilities of your Python scripts by enabling them to interact with web pages broadening the scope of data extraction and allowing for more intricate web scraping operations.
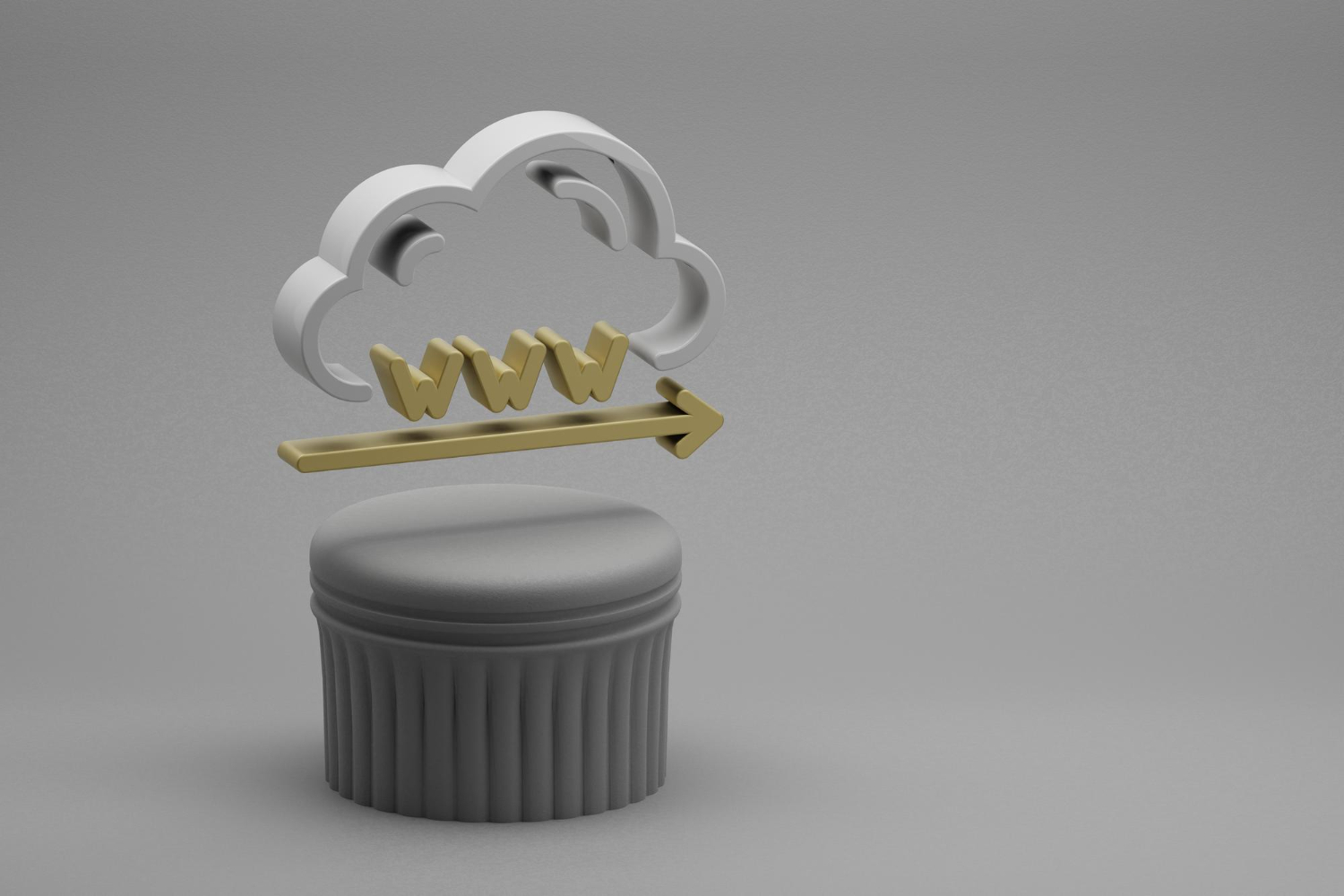
Importing and Using Libraries
Exploring further into the process of importing and utilizing libraries for web scraping in Python involves delving into maximizing the capabilities of these tools. The requests library, essential for handling HTTP requests and BeautifulSoup, a tool, for parsing and navigating HTML content play crucial roles in numerous web scraping endeavors. In this discussion we will delve into applications of these libraries presenting additional code illustrations and providing detailed explanations of their operations.
Advanced Use of ‘requests’
The requests library does more than retrieve the basic HTML data from websites. It provides a solution for managing various types of HTTP requests offering advanced functionalities such as sessions, cookies and headers. These features are crucial, for tackling web scraping tasks.
Handling Sessions and Cookies
Many modern websites use sessions and cookies to manage user interactions. For web scraping, maintaining a session across requests can be crucial for accessing content that requires authentication or preserving a specific state on the website:
with requests.Session() as session:
# Example login procedure
login_url = 'http://example.com/login'
credentials = {'username': 'user', 'password': 'pass'}
session.post(login_url, data=credentials)
# Now, the session is authenticated, subsequent requests will use the same session
profile_url = 'http://example.com/myprofile'
response = session.get(profile_url)
print(response.text) # This would show the profile page of the logged-in user
Customizing Headers
Customizing the request headers can help mimic a real web browser’s behavior more closely, which can be necessary to avoid detection by anti-scraping mechanisms:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get('http://example.com', headers=headers)
print(response.text)
Here, the User-Agent header is set to mimic a popular web browser, which can help in accessing web pages that block requests from non-browser user agents.
Leveraging ‘BeautifulSoup’ for Deeper Data Extraction
While BeautifulSoup simplifies HTML parsing and makes navigating the parse tree intuitive, it also offers powerful features for more complex data extraction tasks.
Extracting Attributes
Sometimes, the data you need is within the attributes of an HTML element (like the href attribute of an <a> tag). BeautifulSoup makes extracting such data straightforward:
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href')) # Prints the URL pointed to by each link
Conditional Data Extraction
‘BeautifulSoup allows for sophisticated searching using attributes, CSS classes, and even text content. This can be particularly useful when you’re looking for specific elements that match certain criteria:
# Find all <a> tags with a specific CSS class
for special_link in soup.find_all('a', class_='special-class'):
print(special_link.text)
# Find elements based on their text content
for heading in soup.find_all('h2', text='Important Heading'):
print(heading.text)
Picking a URL
Before initiating your initial test run, select a URL. Since this web scraping tutorial aims to develop a basic application, we strongly suggest opting for a straightforward target URL:
- Avoid data hidden in Javascript components. They usually require steps to reveal the information you want. Extracting data, from Javascript elements calls for an advanced use of Python and its principles.
- Avoid using image scraping. Selenium allows for downloading images.
- Before you start scraping any data make sure you’re only accessing information that’s publicly available and not violating anyones rights. Also don’t forget to check the robots.txt file, for guidance.
Select the landing page you want to visit and input the URL into the driver.get(‘URL’) parameter. Selenium requires that the connection protocol is provided. As such, it’s always necessary to attach “http://” or “https://” to the URL.
driver.get('https://ipway.com/proxies')
Defining Object and Building Lists
When you start web scraping it’s important to have a plan to manage and save the information you gather from websites. In Python defining objects and creating lists are methods, especially when working with intricate data setups. These approaches go beyond saving data; they help outline how the data connects to real life scenarios and how it can be used and retrieved effectively.
Defining Custom Objects for Data Representation
When you extract information from a website you usually encounter items with characteristics. For example if you’re gathering details about books, from an e commerce site each book could include a title, author, cost and rating. In these situations creating a Python object (or class) offers an organized method to depict each book as a separate entity.
class Book:
def __init__(self, title, author, price, rating):
self.title = title
self.author = author
self.price = price
self.rating = rating
With this Book class, you can create an instance for each book you scrape, with the attributes neatly encapsulated within the object. This not only makes the code cleaner and more maintainable but also makes it easier to work with the data, as you can access each attribute using the dot notation (e.g., book.title).
Utilizing Lists for Dynamic Data Collection
When it comes to dealing with than one item of the same kind individual objects might not cut it. Python lists step in to offer a way to store multiple objects efficiently. In the realm of web scraping lists prove handy, for gathering and structuring data extracted from sources:
# Example of adding a book to the list
new_book = Book("Python Web Scraping", "John Doe", 29.99, 4.5)
books.append(new_book)
# Iterating over the list to print book titles
for book in books:
print(book.title)
Creating custom objects and storing them in lists is an aspect of successful web scraping, in Python. This method provides a level of structure and adaptability allowing you to conveniently handle and retrieve the extracted data. Whether you’re working with a few items or a large number adopting this organized method ensures that your web scraping project is well structured simplifying any future data processing and analysis endeavors.
Extracting Data With a Python Web Scraper
Web scraping at its core involves extracting information, from websites and accomplishing this task using Python demands a mix of accuracy and finesse. The procedure includes pinpointing the data you want to gather exploring the webpages layout to locate this information and utilizing Python scripts to methodically retrieve and save the desired data. Lets delve deeper into these stages to shed light on how a raw HTML file transforms into a organized dataset primed for analysis or additional manipulation.
Identifying Data for Extraction
When starting the extraction process the initial step is to outline the type of information you aim to gather. This may include specifics about products on online stores articles from news websites or property listings on real estate platforms. After determining the data scope the following step involves examining the source code of the web pages that hold this information. Utilizing tools such as Developer Tools, in Chrome or Firefox allows you to explore the HTML layout and pinpoint the tags, attributes and pathways that guide you to the desired data.
Navigating the HTML Structure
Once you know where the data you want is in the HTML layout you can start crafting Python code to move around this layout. This is when tools, like BeautifulSoup become useful. For example if you want to pull out the title of a blog post that’s inside an <h1> tag you’d utilize BeautifulSoup to analyze the HTML file and locate the <h1> tag as follows:
import bs4 from BeautifulSoup
# Assuming 'html_content' contains the HTML source code of the page
soup = BeautifulSoup(html_content, 'html.parser')
blog_title = soup.find('h1').text # Extracts the text within the first <h1> tag found
For web pages with multiple items of the same category (e.g., product listings), you would typically use the find_all method to retrieve all instances of a particular tag:
product_names = [product.text for product in soup.find_all('h2', class_='product-name')]
This particular code snippet locates every tag that has the class product name and gathers the text, within them into a list essentially retrieving the names of all products showcased on the webpage.
Systematic Extraction and Storage
After finding the section in the HTML document and locating the information the next task is to systematically gather this data from, across the website. This could mean going through pages of listings or moving through different parts of a site. The Python requests library can help automate sending HTTP requests to fetch pages while your scraping strategy, defined using BeautifulSoup can extract data from the HTML content of each page.
After extracting the data it’s important to organize it in a manner. Python provides ways to do this such as saving the data in CSV files using the csv module or in Excel files using the pandas library.
import pandas, as pd
# Assuming 'data' is a list of dictionaries containing the scraped data
df = pd.DataFrame(data)
df.to_excel('extracted_data.xlsx', index=False)
Here is an example of how the data can be transformed into a pandas DataFrame and then saved as an Excel file making it easier to organize and access the information collected.
Exporting the data to CSV
Transferring the collected and organized data into a CSV (Comma Separated Values) file is an essential task in web scraping endeavors. This standard format is widely.
Can be smoothly integrated into different data analysis tools, databases and spreadsheet programs providing a flexible option for storing and distributing scraped information. Now lets explore a method for effectively exporting the results of your Python web scraper to a CSV file guaranteeing that your data stays preserved and available, for future needs.
- Preparing the Data for Export
- Utilizing Python’s ‘csv’ Module
Python’s built-in csv module provides the necessary functionality to write your structured data to a CSV file with minimal hassle. To start, you’ll need to import the module and prepare to write to a file:
import csv
# Assuming 'data' is your list of dictionaries
data = [{'name': 'Product 1', 'price': '19.99', 'description': 'A product description'},
{'name': 'Product 2', 'price': '29.99', 'description': 'Another product description'}]
# Define the CSV file name
filename = 'exported_data.csv'
3. Writing to a CSV File
With your data ready and the csv module imported, the next step is to open a new CSV file in write mode and use a csv.DictWriter object to write the data. The DictWriter is particularly suited for handling lists of dictionaries, as it maps each dictionary onto a row in the CSV file, with the dictionary keys automatically used as column headers:
# Specify the fieldnames based on the dictionary keys
fieldnames = ['name', 'price', 'description']
with open(filename, mode='w', newline='', encoding='utf-8') as file:
writer = csv.DictWriter(file, fieldnames=fieldnames)
# Write the header row
writer.writeheader()
# Write the data rows
for item in data:
writer.writerow(item)
This code snippet creates a CSV file called exported_data.csv. It first writes the header row. Then adds each item from the data list. The newline=” parameter is used to prevent newline characters from being added between rows, in the CSV file and encoding=’utf 8′ ensures that the file can support various characters safeguarding your data’s integrity.
Exporting the data to Excel
Moving the collected information from your Python web scraping project to an Excel spreadsheet enhances its usefulness providing an adaptable platform for analyzing, visualizing and presenting data.
Excels popularity in business and education makes it a suitable choice, for sharing extracted data enabling parties to explore the findings without dealing with the intricacies of web scraping. This section is designed to walk you through the process of transferring your scraped data to an Excel document focusing on web scraping tools used by Python developers to ensure that the data remains well structured and easily accessible.
- Structuring Your Data for Excel Export
- Leveraging ‘pandas’ for Excel Export
The Python pandas library is well known for its data manipulation abilities and it also offers simple ways to export data to Excel. This functionality comes in handy especially when working on Python web scraping tasks as it makes the process of moving from scraped data to an organized Excel file much easier. If you don’t have pandas installed yet you can easily add it using pip.
pip install pandas
With pandas installed, you can proceed to import it into your script and prepare your data for export:
import pandas as pd
# Assuming 'data' is your list of dictionaries from the scraping process
data_frame = pd.DataFrame(data)
- Exporting to an Excel File
Once your data is encapsulated within a DataFrame, exporting it to an Excel file is a matter of calling a single method:
# Define the Excel file name
excel_filename = 'scraped_data.xlsx'
# Use the to_excel method to write the DataFrame to an Excel file
data_frame.to_excel(excel_filename, index=False)
- Enhancing Your Web Scraping Project’s Deliverables
Web Scraping Python – Best Practices
When using web scraping with Python, it’s crucial to approach this data extraction method with caution to ensure efficiency, legality and respect for the websites you’re targeting. Following recommended practices not protects your scraping efforts but also upholds the integrity and accessibility of online content. As you dive into web scraping endeavors following these guidelines will improve your workflow and results.
- Respect Robots Exclusion Protocol: Make sure to review the robots.txt file before you scrape any website. You can usually locate it at the root directory like http;//example.com/robots.txt. It outlines the areas of the site that web crawlers should steer clear of. Following these rules is crucial, for scraping practices and to prevent getting your IP blocked.
- Throttle Your Requests: Excessive rapid requests to a website may overwhelm its server leading to service interruptions. To prevent this adopt a crawling approach by interspersing requests, with breaks using time intervals (time.sleep()). By imitating browsing patterns in this manner you can reduce the likelihood of being identified as a web scraper.
import time
# Pause for 1 second between requests
time.sleep(1)
- Use Headers and Rotate User-Agents: Make sure to specify your web scraper by adding a User Agent header in your requests. This openness can occasionally help avoid getting blocked while scraping. Additionally changing User Agent strings can simulate browsers and devices making your scraping actions look more, like normal web traffic.
import requests
headers = {
'User-Agent': 'Your Web Scraper Name/Version',
}
response = requests.get('http://example.com', headers=headers)
- Engage in Ethical Scraping: Remember to think about how your scraping might affect the website you’re targeting. Steer clear of scraping information from sites that clearly state its not allowed in their terms of service. If you’re unsure reaching out to the website owner can help you understand if they are okay, with your scraping efforts.
- Opt for API Use When Available: Numerous websites provide APIs that allow users to access their data. It is advisable to utilize these APIs whenever available as they tend to be more effective, dependable and considerate of the websites data and limitations. Additionally APIs often present data in a manner lessening the requirement, for intricate parsing algorithms.
Conclusion
In summary becoming skilled, in web scraping using Python unlocks a range of opportunities for data enthusiasts, researchers and professionals in fields. By following the step by step instructions provided in this article starting from setting up your Python environment and choosing the libraries to effectively extracting and exporting data you are ready to leverage the potential of web data.
The process of defining objects navigating HTML structures and applying recommended methods sheds light on the journey to mastering web scraping. As you begin this adventure keep in mind that the true value lies not in gathering data but, in translating that data into practical insights.
Discover how IPWAY’s innovative solutions can revolutionize your web scraping experience for a better and more efficient approach.