Web scraping with Java has become a method in todays digital world as data plays a significant role in various sectors. This method entails extracting information, from websites offering a wide range of uses from conducting market studies to monitoring real time data.
Java renowned for its libraries and ability to work across different platforms provides a strong base for creating web scraping tools. This guide about web scraping with Java explores the intricacies of web scraping with Java showcasing frameworks such as JSoup and HtmlUnit to illustrate efficient data retrieval techniques. Whether you’re just starting out or a developer mastering the art of using Java for web scraping can open up valuable avenues for gaining insights, from data.
Web Scraping Frameworks
Two of the primary libraries utilized for web scraping with Java are JSoup and HtmlUnit.
JSoup is known for its capabilities, in managing poorly structured HTML efficiently. Its name comes from “tag soup,” which refers to organized HTML files.
HtmlUnit however functions as a browser without a graphical user interface designed specifically for Java programs. It imitates browser features such as fetching elements and clicking which makes it essential for conducting unit tests as its name suggests. This tool acts as a way to simulate browser actions, for testing objectives.
Furthermore HtmlUnit is useful for web scraping. It provides an option to easily disable JavaScript and CSS with just one command, which is beneficial, for scraping projects that do not require these components. In the following sections we will explore both libraries. Create web scrapers.
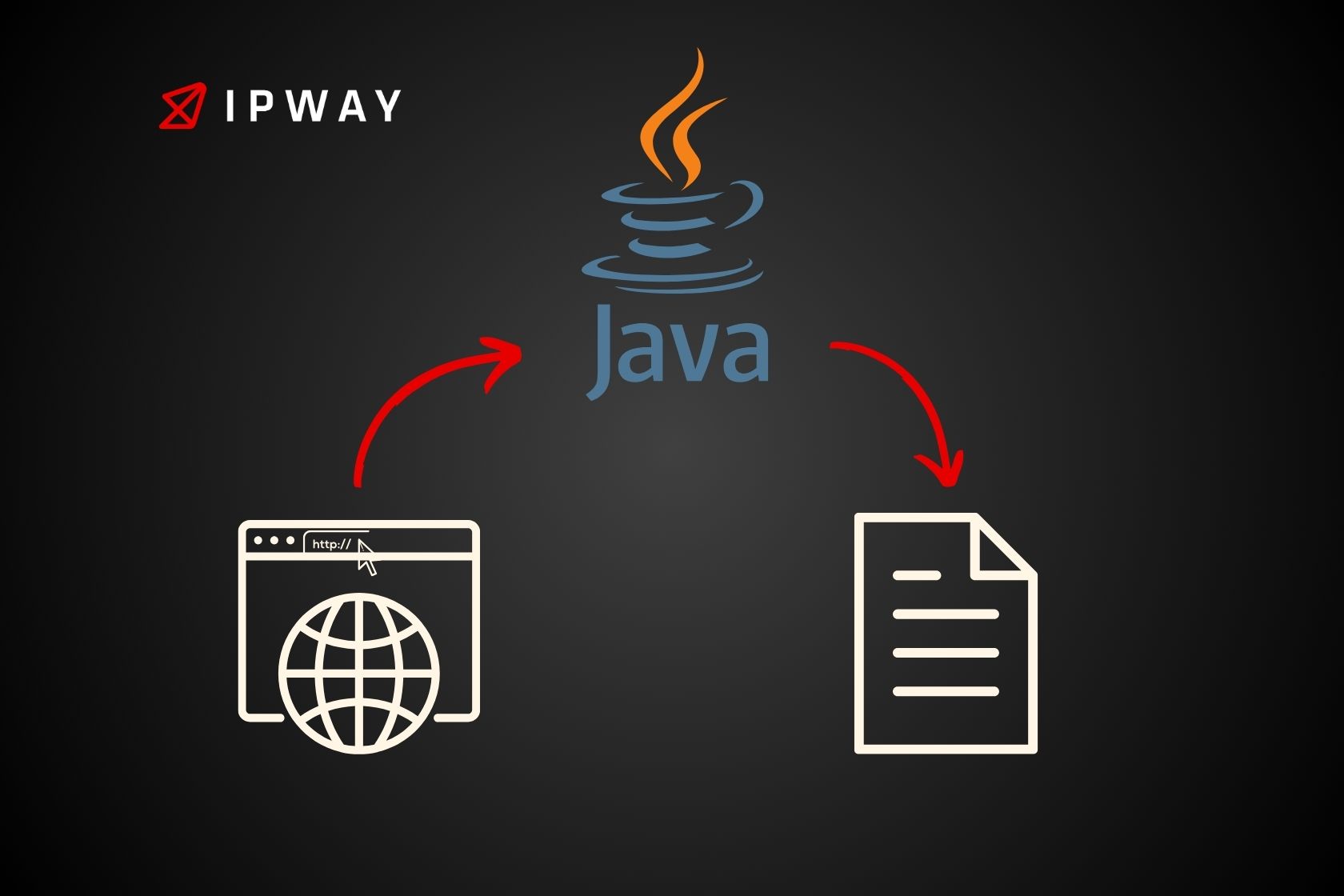
Prerequisite for building web scraping with Java
To start web scraping with Java, you need a basic setup:
Java Development Kit (JDK): Make sure you’ve got the most up, to date JDK installed on your computer to make the most of Javas capabilities.
Integrated Development Environment (IDE): Using tools, like IntelliJ IDEA, Eclipse or NetBeans can make coding much easier for you.
Maven or Gradle: Here are some tools that can assist you in organizing your projects requirements and setting up its structure.
Getting Started
Before delving into the details of using Java for web scraping it’s important to lay a strong groundwork by configuring your Java development environment and project. This segment will walk you through the procedures needed to kickstart your web scraping with Java.
Setting Up Your Java Development Environment
To begin Java development you’ll have to install the Java Development Kit (JDK) and an Integrated Development Environment (IDE). Here’s a guide, on how to get them up and running:
Download and Install JDK: Head over to the Oracle website. Grab the most recent JDK version. Follow the installation steps tailored for your operating system.
Choose and Install an IDE: When it comes to Java development there are IDE options available such, as IntelliJ IDEA, Eclipse and NetBeans. You can choose the one that suits your requirements best by downloading and installing it. For those starting out IntelliJ IDEA or Eclipse are often suggested because of their community backing and wide range of plugins.
Set Up JDK in Your IDE: Once you’ve set up your IDE make sure to adjust the settings to connect it with the JDK you installed. You can usually find this option in either the project settings or system preferences, within your IDE.
Creating Your First Java Project
After setting up your development environment the next task is to establish a Java project and include the required dependencies, for web scraping.
Create a New Java Project:
- In IntelliJ IDEA: Go to File -> New -> Project, select Java from the left panel, and click Next. Follow the prompts to configure your project settings
- In Eclipse: Go to File -> New -> Java Project. Enter a project name and click Finish.
Add Dependencies:
- Maven: If you are using Maven, add the dependencies for JSoup and HtmlUnit to your pom.xml file
<dependencies>
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>1.13.1</version>
</dependency>
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit</artifactId>
<version>2.40.0</version>
</dependency>
</dependencies>
- Gradle: If using Gradle, add the dependencies in your build.gradle file:
dependencies {
implementation 'org.jsoup:jsoup:1.13.1'
implementation 'net.sourceforge.htmlunit:htmlunit:2.40.0'
}
Write a Simple Scraper
Here is a simple illustration of utilizing JSoup to retrieve and interpret HTML content from a webpage. You can insert this code into the function of your main program.
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.select.Elements;
public class Main {
public static void main(String[] args) {
try {
Document doc = Jsoup.connect("http://example.com").get();
Elements paragraphs = doc.select("p");
paragraphs.forEach(paragraph -> System.out.println(paragraph.text()));
} catch (IOException e) {
e.printStackTrace();
}
}
}
Web Scraping With Java Using JSoup
JSoup is a Java library that is user friendly and crafted for handling real world HTML tasks. It offers an interface for retrieving and managing data incorporating elements from DOM, CSS and jQuery methods. In this segment we will delve into the basics of utilizing JSoup for web scraping purposes showcasing its functionalities with, in depth illustrations.
Introduction to JSoup
Developers using JSoup in Java can parse HTML documents from sources such, as URLs, files or strings. The tool enables them to locate and extract data through DOM traversal or CSS selectors. JSoup stands out for its flexibility and ability to handle HTML structures effectively. It automatically cleans up the HTML during parsing ensuring an efficient data extraction process.
Setting Up Your JSoup in Your Project
First, you need to include JSoup in your project. If you are using Maven, add the following dependency to your pom.xml:
<dependency>
<groupId>org.jsoup</groupId>
<artifactId>jsoup</artifactId>
<version>1.13.1</version>
</dependency>
For Gradle users, add this line to your build.gradle:
implementation 'org.jsoup:jsoup:1.13.1'
Fetching and Parsing HTML with JSoup
To start extracting information, from websites you must. Analyze an HTML file. Here’s how you can do it with JSoup:
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
public class WebScraper {
public static void main(String[] args) {
String url = "https://example.com";
try {
// Fetch the HTML code
Document document = Jsoup.connect(url).get();
System.out.println("Title: " + document.title());
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this instance the Jsoup.connect(url).get() function is employed to fetch the HTML content, from the provided URL. After retrieving the document you have the ability to utilize JSoups parsing features to interact with sections of the webpage.
Extracting Data Using Colectors
You can utilize the function, in JSoup to employ CSS style selectors for locating and retrieving information from the HTML file. Here’s an example of extracting all hyperlinks from a webpage:
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
public class LinkExtractor {
public static void main(String[] args) {
try {
Document document = Jsoup.connect("https://example.com").get();
Elements links = document.select("a[href]"); // a with href attribute
for (Element link : links) {
System.out.println("Link: " + link.attr("abs:href"));
System.out.println("Text: " + link.text());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code snippet, when you use document.select(“a[href]”) it fetches all tags that have an href attribute (meaning they are links). After that the loop displays both the URL and the text of each link.
Handling Complex Data Extraction
JSoup is skilled at dealing with situations like extracting information, from JavaScript code or uncovering data concealed within HTML element attributes. Suppose you want to scrape dynamic content generated by JavaScript:
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
public class DynamicContentScraper {
public static void main(String[] args) {
try {
Document document = Jsoup.connect("https://example.com").get();
Element scriptElement = document.select("script#data").first();
String jsonData = scriptElement.data();
System.out.println("Script Data: " + jsonData);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Here, document.select(“script#data”).first() selects the first <script> tag with an ID of “data”, and scriptElement.data() gets the data inside the script tag, which might be JSON or other JavaScript objects.
Web Scraping With Java Using HTML Unit
HtmlUnit is a Java tool that functions as a browser without a visible interface making it great for mimicking how a user browses the web. It’s especially handy for tasks like web scraping where interaction with JavaScript pages is necessary.
HtmlUnit can run JavaScript in the background manage AJAX requests and replicate actions such as clicks, form submissions and navigation like an actual browser. In this part we’ll explore using HtmlUnit for web scraping in detail by showcasing its capabilities, with specific examples.
Introduction to HTML Unit
HtmlUnit is a Java tool that functions as a headless browser perfect for mimicking a users online navigation. It comes in handy for tasks like web scraping where interaction with JavaScript web pages is crucial.
HtmlUnit can run JavaScript in the background manage AJAX requests and replicate actions such as clicks, form submissions and page navigation just as if it were a person browsing. This section will explore HtmlUnits usage in web scraping further by showcasing its capabilities and practicality, with examples.
Setting Up Your HTML Unit in Your Project
To incorporate HtmlUnit into your Java project, you must first add the necessary dependencies. If you are using Maven, include the following in your pom.xml file:
<dependency>
<groupId>net.sourceforge.htmlunit</groupId>
<artifactId>htmlunit</artifactId>
<version>2.40.0</version>
</dependency>
For Gradle users, the dependency line in your build.gradle will look like this:
implementation 'net.sourceforge.htmlunit:htmlunit:2.40.0'
Creating a WebClient Instance
The first step in using HtmlUnit is to create an instance of WebClient, which represents a browser:
mport com.gargoylesoftware.htmlunit.WebClient;
public class WebClientExample {
public static void main(String[] args) {
try (WebClient webClient = new WebClient()) {
// Configure the webClient according to your needs
webClient.getOptions().setCssEnabled(false); // if not interested in CSS
webClient.getOptions().setJavaScriptEnabled(true); // if you need JavaScript support
}
}
}
Navigating Web Pages
Using HtmlUnit allows you to browse web pages like a regular user would, with a browser. Here’s how to load a page and access its title:
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
public class NavigationExample {
public static void main(String[] args) {
try (WebClient webClient = new WebClient()) {
HtmlPage page = webClient.getPage("http://example.com");
System.out.println("Page Title: " + page.getTitleText());
} catch (Exception e) {
e.printStackTrace();
}
}
}
Interacting with Pages
You can use HtmlUnit to engage with components, on a web page like completing forms and pressing buttons. Here is an example of how to submit a form:
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlForm;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
import com.gargoylesoftware.htmlunit.html.HtmlSubmitInput;
import com.gargoylesoftware.htmlunit.html.HtmlTextInput;
public class FormInteractionExample {
public static void main(String[] args) {
try (WebClient webClient = new WebClient()) {
HtmlPage page = webClient.getPage("http://example.com/formPage");
HtmlForm form = page.getFormByName("myForm");
HtmlTextInput textField = form.getInputByName("textFieldName");
textField.setValueAttribute("test value");
HtmlSubmitInput submitButton = form.getInputByName("submitButtonName");
HtmlPage responsePage = submitButton.click();
System.out.println("Response Page Title: " + responsePage.getTitleText());
} catch (Exception e) {
e.printStackTrace();
}
}
}
This example demonstrates how to locate a form, fill in text, and submit it to see the response.
Handling Complex JavaScript and AJAX
HtmlUnit excels in managing JavaScript and AJAX driven websites. When HtmlUnit loads a page it runs the JavaScript as if it were a browser, crucial, for extracting dynamic content.
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
public class JavaScriptHandlingExample {
public static void main(String[] args) {
try (WebClient webClient = new WebClient()) {
webClient.getOptions().setJavaScriptEnabled(true);
HtmlPage myPage = webClient.getPage("http://example.com/dynamicContent");
// Assuming there's a delay in loading content
webClient.waitForBackgroundJavaScript(10000); // wait up to 10 seconds
System.out.println("Page Content: " + myPage.asText());
} catch (Exception e) {
e.printStackTrace();
}
}
}
This sample sets up the WebClient to allow JavaScript and wait for any background JavaScript processes, such, as AJAX calls to complete.
Conclusion
Using web scraping with Java is an approach to extract data suitable for dealing with various levels of complexity. By utilizing tools such as JSoup and HtmlUnit programmers can create scrapers that are capable of browsing websites and collecting data from them. With the guidance given you now have the understanding to begin web scraping with Java efficiently. Whether you’re interested in analyzing data gaining insights or automating tests Java offers a dependable framework, for your web scraping requirements.
Web scraping with Java involves more, than technical aspects—it requires a deep understanding of data organization and ethical considerations.
Discover how IPWAY’s innovative solutions can revolutionize your web scraping experience for a better and more efficient approach.